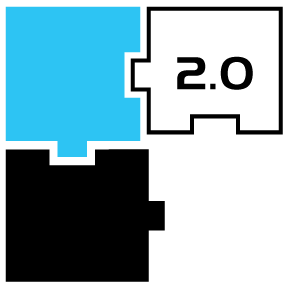 |
mikroSDK Reference Manual
|
Image Drawing API.
More...
This API is used for drawing and managing images on the display.
◆ gl_draw_image()
This function draws an image on display. It is specialized for drawing support image formats, but may redefined by user. For that option please have a look at the Image Graphics Format Handlers.
- Parameters
-
[in] | dest | Frame in which the image will be drawn. See gl_rectangle_t structure definition for detailed explanation. |
[in] | src | Part of the original image which will be drawn into dest frame. See gl_rectangle_t structure definition for detailed explanation. |
[in] | image | Pointer to an image. |
- Returns
- Returns zero if the image is successfully drawn, otherwise returns non-zero value.
- Precondition
- Before using this function a driver must be set using the gl_set_driver function.
- Note
- Image must be generated by NECTO Studio's resource generator.
- See also
- gl_draw_jpeg_image, gl_draw_bitmap_16bpp, gl_draw_bitmap_8bpp, gl_draw_bitmap_4bpp, gl_draw_bitmap_1bpp
Example
#include "board.h"
#include "tft8.h"
#include "resource.h"
static tft8_cfg_t tft_cfg;
void main()
{
TFT8_MAP_PINOUTS_16BIT(tft_cfg);
tft_cfg.board = &TFT_BOARD_7_CAPACITIVE;
tft8_init(&tft_cfg, &driver);
{
}
{
}
}
◆ gl_image_width()
uint16_t gl_image_width |
( |
const uint8_t *__generic |
image | ) |
|
This function returns the width of the image.
- Parameters
-
[in] | image | Pointer to an image. |
- Returns
- If the image is not null returns width of the image, otherwise returns zero.
- Note
- Image must be generated by NECTO Studio's resource generator.
Example
#include "board.h"
#include "tft8.h"
#include "resource.h"
static tft8_cfg_t tft_cfg;
const code char* images[] = {
mikroejpg,
mikroebmp1,
mikroebmp4,
mikroebmp8,
mikroebmp16
};
char str_buff[10];
}
void main()
{
TFT8_MAP_PINOUTS_16BIT(tft_cfg);
tft_cfg.board = &TFT_BOARD_7_CAPACITIVE;
tft8_init(&tft_cfg, &driver);
for (int i = 0; i < 5 ; i++)
{
}
}
◆ gl_image_height()
uint16_t gl_image_height |
( |
const uint8_t *__generic |
image | ) |
|
This function returns the height of the image.
- Parameters
-
[in] | image | Pointer to an image. |
- Returns
- If the image is not null returns height of the image, otherwise returns zero.
- Note
- Image must be generated by NECTO Studio's resource generator.
Example
#include "board.h"
#include "tft8.h"
#include "resource.h"
static tft8_cfg_t tft_cfg;
const code char* images[] = {
mikroejpg,
mikroebmp1,
mikroebmp4,
mikroebmp8,
mikroebmp16
};
char str_buff[10];
}
void main()
{
TFT8_MAP_PINOUTS_16BIT(tft_cfg);
tft_cfg.board = &TFT_BOARD_7_CAPACITIVE;
tft8_init(&tft_cfg, &driver);
for (int i = 0; i < 5 ; i++)
{
}
}
◆ gl_image_format()
This function returns the format of the image.
- Parameters
-
[in] | image | Pointer to an image. |
- Returns
- If the image is not null returns format of image, otherwise returns zero. See gl_image_format_t structure definition for detailed explanation.
- Note
- Image must be generated by NECTO Studio's resource generator.
Example
#include "board.h"
#include "tft8.h"
static tft8_cfg_t tft_cfg;
extern const code char mikroejpg[2701];
extern const code char mikroebmp1[2662];
extern const code char mikroebmp4[10493];
extern const code char mikroebmp8[21428];
extern const code char mikroebmp16[41826];
void main()
{
TFT8_MAP_PINOUTS_16BIT(tft_cfg);
tft_cfg.board = &TFT_BOARD_7_CAPACITIVE;
tft8_init(&tft_cfg, &driver);
else
else
else
else
else
}
uint16_t width
Definition: gl_types.h:131
Definition: gl_types.h:74
void uint16_to_str(uint16_t input, char *output)
Converts uint16_t to string.
void gl_set_font(const uint8_t *font)
Initialize the active font.
The context structure for storing driver configuration.
Definition: gl_types.h:145
void gl_set_brush_color(gl_color_t color)
Sets active brush color.
Definition: gl_types.h:76
Definition: gl_colors.h:147
Definition: gl_colors.h:212
gl_point_t top_left
Definition: gl_types.h:130
uint16_t gl_image_width(const uint8_t *__generic image)
Calculate width of the image.
Definition: gl_colors.h:246
void gl_draw_text(const char *__generic text, gl_coord_t x, gl_coord_t y)
Write a text on display.
void gl_set_pen_width(uint16_t width)
Sets the active pen width.
uint16_t height
Definition: gl_types.h:132
void gl_set_driver(gl_driver_t *driver)
Sets the driver to the active state and enables drawing on whole display.
Conversion function prototypes.
The context structure for storing rectangle by its top left point and width and height (in pixels).
Definition: gl_types.h:128
Definition: gl_types.h:73
uint16_t gl_image_height(const uint8_t *__generic image)
Calculate height of the image.
int gl_draw_image(gl_rectangle_t *dest, gl_rectangle_t *src, const uint8_t *__generic image)
Draw image on display.
Definition: gl_types.h:75
int16_t gl_coord_t
Definition: gl_types.h:102
void gl_clear(gl_color_t color)
Paint whole display.
Definition: gl_types.h:77
gl_image_format_t gl_image_format(const uint8_t *__generic image)
Return format of the image.
gl_int_t y
Definition: gl_types.h:111
uint16_t gl_uint_t
Definition: gl_types.h:91
gl_int_t x
Definition: gl_types.h:110
void gl_draw_circle(gl_coord_t x0, gl_coord_t y0, gl_uint_t radius)
Draw a circle on display.