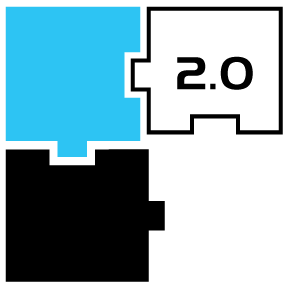 |
mikroSDK Reference Manual
|
Graphics Library API reference.
More...
API for configuring and manipulating Graphics library.
Example
#include "board.h"
#include "tft8.h"
static tft8_cfg_t tft_cfg;
void main()
{
TFT8_MAP_PINOUTS_16BIT(tft_cfg);
tft_cfg.board = &TFT_BOARD_7_CAPACITIVE;
tft8_init(&tft_cfg, &driver);
}
Definition: gl_types.h:61
Definition: gl_colors.h:101
The context structure for storing driver configuration.
Definition: gl_types.h:145
void gl_set_brush_color(gl_color_t color)
Sets active brush color.
Definition: gl_colors.h:147
void gl_draw_rect(gl_coord_t top_left_x, gl_coord_t top_left_y, gl_uint_t width, gl_uint_t height)
Draw a rectangle on display.
Definition: gl_colors.h:238
void gl_set_brush_color_from(gl_color_t color)
Sets the active start color.
Definition: gl_colors.h:197
Definition: gl_colors.h:207
void gl_set_pen_width(uint16_t width)
Sets the active pen width.
uint16_t gl_get_screen_height()
Returns the height of the display.
void gl_set_driver(gl_driver_t *driver)
Sets the driver to the active state and enables drawing on whole display.
void gl_draw_line(gl_coord_t x1, gl_coord_t y1, gl_coord_t x2, gl_coord_t y2)
Draw a line on the display.
uint16_t gl_get_screen_width()
Returns the width of the display.
bool gl_set_crop_borders(gl_coord_t left, gl_coord_t right, gl_coord_t top, gl_coord_t bottom)
Initialize borders for drawing on display.
void gl_set_brush_style(gl_brush_style_t style)
Sets active brush style.
void gl_clear(gl_color_t color)
Paint whole display.
void gl_set_brush_color_to(gl_color_t color)
Sets the active end color.
Definition: gl_types.h:60
void gl_draw_rect_rounded(gl_coord_t top_left_x, gl_coord_t top_left_y, gl_uint_t width, gl_uint_t height, gl_uint_t radius)
Draw a rounded rectangle on display.
Definition: gl_colors.h:165
void gl_set_pen(gl_color_t color, uint16_t width)
Sets the active pen width and color.
Definition: gl_colors.h:99
void gl_draw_circle(gl_coord_t x0, gl_coord_t y0, gl_uint_t radius)
Draw a circle on display.
Definition: gl_colors.h:92