PWM Motor Control Library
The PWM Motor Control module is available with a number of dsPIC30/33 MCUs. The mikroC PRO for dsPIC30/33 and PIC24 provides a library which
simplifies using the PWM Motor Control module.
Important :
- Number of PWM modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
- Where applicable, PWM library routines require you to specify the module you want to use. To use the desired PWM module, simply change the letter x in the routine prototype for a number from 1 to 2.
Library Routines
PWMx_Mc_Init
Prototype |
(I) unsigned int PWMx_Mc_Init(unsigned long freq_hz, unsigned int pair_output_mode, unsigned int enable_channel, unsigned int clock_prescale_output_postscale);
(II) unsigned int PWM1_MC_Init(unsigned long freq_hz, unsigned int pair_output_mode, unsigned int enable_output_x, unsigned int clock_prescale_output_postscale);
(III) unsigned int PWM_MC_Init(unsigned long freq_hz, unsigned primary, unsigned clock_prescale);
|
Description |
Initializes the Motor Control PWM module with duty ratio 0.
The function calculates timer period, writes it to the MCU's PTPER register and returns it as the function result.
|
Parameters |
(I)
freq_hz: PWM frequency in Hz (refer to device datasheet for correct values in respect with Fosc).
pair_output_mode: output mode for output pin pairs. Valid values are given in the table below :
Value |
Description |
_PWM_MC_CH1_PAIR_INDEPENDENT_MODE |
PWM1L and PWM1H will in independent mode. |
_PWM_MC_CH1_PAIR_COMPLEMENTARY_MODE |
PWM1L and PWM1H will in complementary mode. |
_PWM_MC_CH2_PAIR_INDEPENDENT_MODE |
PWM2L and PWM2H will in independent mode. |
_PWM_MC_CH2_PAIR_COMPLEMENTARY_MODE |
PWM2L and PWM2H will in complementary mode. |
_PWM_MC_CH3_PAIR_INDEPENDENT_MODE |
PWM3L and PWM3H will in independent mode. |
_PWM_MC_CH3_PAIR_COMPLEMENTARY_MODE |
PWM3L and PWM3H will in complementary mode. |
_PWM_MC_CH4_PAIR_INDEPENDENT_MODE |
PWM4L and PWM4H will in independent mode. |
_PWM_MC_CH4_PAIR_COMPLEMENTARY_MODE |
PWM4L and PWM4H will in complementary mode. |
enable_channel: enable PWM channel. Valid values are given in the table below :
Value |
Description |
_PWM_MC_CH1L |
Enable PWM1L channel. |
_PWM_MC_CH2L |
Enable PWM2L channel. |
_PWM_MC_CH3L |
Enable PWM3L channel. |
_PWM_MC_CH4L |
Enable PWM4L channel. |
_PWM_MC_CH1H |
Enable PWM1H channel. |
_PWM_MC_CH2H |
Enable PWM2H channel. |
_PWM_MC_CH3H |
Enable PWM3H channel. |
_PWM_MC_CH4H |
Enable PWM4H channel. |
clock_prescale_output_postscale: PWM clock prescaler/postscaler settings. Values <0..3> and <0..15> correspond to prescaler/postscaler <1:1, 1:4, 1:16, 1:64> and <1:1, 1:2, ..., 1:16>
(II)
freq_hz: PWM frequency in Hz (refer to device datasheet for correct values in respect with Fosc)
pair_output_mode: output mode for output pin pairs: 1 = independent, 0 = complementary (for dsPIC33EPXXGS50X, dsPIC33EPXXGS202 and dsPIC33EVXXXGM00X_10X 0 = independent, 1 = complementary)
If pair_output_mode.B0 is equal to 1 then PWM channels PWM1L and PWM1H will be independent (for dsPIC33EPXXGS50X, dsPIC33EPXXGS202 and dsPIC33EVXXXGM00X_10X complementary),
If pair_output_mode.B1 is equal to 0 then PWM channels PWM2L and PWM2H will be complementary (for dsPIC33EPXXGS50X, dsPIC33EPXXGS202 and dsPIC33EVXXXGM00X_10X independent),
...
If pair_output_mode.Bn is equal to 1 then PWM channels PWM(n+1)L and PWM(n+1)H will be independent (for dsPIC33EPXXGS50X, dsPIC33EPXXGS202 and dsPIC33EVXXXGM00X_10X complementary),
If pair_output_mode.Bn is equal to 0 then PWM channels PWM(n+1)L and PWM(n+1)H will be complementary (for dsPIC33EPXXGS50X, dsPIC33EPXXGS202 and dsPIC33EVXXXGM00X_10X independent).
enable_output_x: bits <7..0> are enabling corresponding PWM channels (PWM4H, PWM3H, PWM2H, PWM1H, PWM4L, PWM3L, PWM2L, PWM1L ).
(for dsPIC33EPXXGS50X, dsPIC33EPXXGS202 and dsPIC33EVXXXGM00X_10X PWM5H, PWM5L, PWM4H, PWM4L, PWM3H, PWM3L, PWM2H, PWM2L, PWM1H, PWM1L)
If bit value is equal to 0 then corresponding PWM channel is disabled (pin is standard I/O).
If bit value is equal to 1 then corresponding PWM channel is enabled (pin is PWM output).
For detalied explanation consult the "Motor Control PWM Module" section in device datasheet.
clock_prescale_output_postscale: PWM clock prescaler/postscaler settings. Values <0..3> and <0..15>
correspond to prescaler/postscaler <1:1, 1:4, 1:16, 1:64> and <1:1, 1:2, ..., 1:16>
(III)
freq_hz: PWM frequency in Hz (refer to device datasheet for correct values in respect with Fosc).
primary : chose beetween primary and secondary clock register, valid values :
Value |
Description |
_PWM_MC_CLK_PRIMARY |
Primary clock register. |
_PWM_MC_CLK_SECONDARY |
Secondary clock register. |
clock_prescale: PWM clock prescaler settings.
|
Returns |
Calculated timer period.
|
Requires |
The dsPIC30/33 MCU must have the Motor Control PWM module.
|
Example |
// Initializes the PWM1 module at 5KHz, complementary pin-pair output, output enabled on pins 4l..1l, no clock prescale and no clock postscale:
unsigned int duty_50;
...
duty_50 = PWM1_Mc_Init(5000, 1, 0x0F, 0);
|
Notes |
- Number of PWM modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
- PWM library routines require you to specify the module you want to use. To use the desired PWM module, simply change the letter x in the routine prototype for a number from 1 to 2.
|
PWMx_Mc_Set_Duty
Prototype |
void PWM1_Mc_Set_Duty(unsigned duty, unsigned channel);
// For dsPIC 33FJ MCUs that have PWM2 module :
void PWM2_Mc_Set_Duty(unsigned duty);
void PWM_MC_Set_Duty(unsigned duty, unsigned primary, unsigned channel);
|
Description |
The function changes PWM duty ratio.
|
Parameters |
duty: PWM duty ratio. Valid values: 0 to timer period returned by the PWMx_Mc_Init function.
channel: number of PWM channel to change duty to.
primary : chose beetween primary and secondary clock register.
Value |
Description |
_PWM_MC_DUTY_PRIMARY |
Primary duty cycle register. |
_PWM_MC_DUTY_SECONDARY |
Secondary duty cycle register. |
|
Returns |
Nothing.
|
Requires |
The dsPIC30/33 MCU must have the Motor Control PWM module.
The PWM module needs to be initalized. See the PWMx_Mc_Init function.
|
Example |
// Set duty ratio to 50% at channel 1:
PWM1_Mc_Init(5000,1,0xF,0);
...
PWM1_Mc_Set_Duty(32767, 1);
|
Notes |
- Number of PWM modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
- PWM library routines require you to specify the module you want to use. To use the desired PWM module, simply change the letter x in the routine prototype for a number from 1 to 2.
|
PWM_MC_Set_Master_Duty
Prototype |
void PWM_MC_Set_Master_Duty(unsigned duty);
|
Description |
This function updates master duty cycle register.
|
Parameters |
duty: PWM duty ratio. Valid values: 0 to timer period returned by the PWMx_Mc_Init function.
|
Returns |
Nothing.
|
Requires |
The PWM module needs to be initalized. See the PWMx_Mc_Init function.
|
Example |
// Set duty ratio to 50% :
PWM_MC_Set_Master_Duty(32768);
|
Notes |
- This routine is avaliable only for dsPIC33EPXXX(GP/MC/MU)806/810/814 and PIC24EPXXX(GP/GU)810/814 family.
|
PWMx_Mc_Start
Prototype |
void PWMx_Mc_Start();
|
Description |
Starts the Motor Control PWM module (channels initialized in the PWMx_Mc_Init function).
|
Parameters |
None.
|
Returns |
Nothing.
|
Requires |
The dsPIC30/33 MCU must have the Motor Control PWM module.
The PWM module needs to be initalized. See the PWMx_Mc_Init function.
|
Example |
// start the Motor Control PWM1 module
PWM1_Mc_Start();
|
Notes |
- Number of PWM modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
- PWM library routines require you to specify the module you want to use. To use the desired PWM module, simply change the letter x in the routine prototype for a number from 1 to 2.
|
PWMx_Mc_Stop
Prototype |
void PWMx_Mc_Stop();
|
Description |
Stops the Motor Control PWM module.
|
Parameters |
None.
|
Returns |
Nothing.
|
Requires |
The dsPIC30/33 MCU must have the Motor Control PWM module.
|
Example |
// stop the Motor Control PWM1 module
PWM1_Mc_Stop();
|
Notes |
- Number of PWM modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
- PWM library routines require you to specify the module you want to use. To use the desired PWM module, simply change the letter x in the routine prototype for a number from 1 to 2.
|
PWM_MC_Init_Channel
Prototype |
void PWM_MC_Init_Channel(unsigned pinEnableMask, unsigned mode, unsigned clk, unsigned masterDutyCycle, unsigned channel);
|
Description |
This function initializes single PWM channel.
|
Parameters |
pinEnableMask: PWM Output Pin Ownership bit, valid values :
Value |
Description |
_PWM_MC_ENABLE_LOW_PIN |
PWM module controls PWMxL pin. |
_PWM_MC_ENABLE_HIGH_PIN |
PWM module controls PWMxH pin. |
mode: PWM I/O Pin Mode bits, valid values :
Value |
Description |
_PWM_MC_TRUE_INDEPENDENT |
PWM I/O pin pair is in the True Independent Output mode. |
_PWM_MC_PUSH_PULL |
PWM I/O pin pair is in the Push-Pull Output mode. |
_PWM_MC_REDUNDANT |
PWM I/O pin pair is in the Redundant Output mode. |
_PWM_MC_COMPLEMENTARY |
PWM I/O pin pair is in the Complementary Output mode. |
primary: choose beetween primary and secondary clock register, valid values :
Value |
Description |
_PWM_MC_CLK_PRIMARY |
Primary clock register. |
_PWM_MC_CLK_SECONDARY |
Secondary clock register. |
masterDutyCycle: Enable/disable master duty cycle register, valid values :
Value |
Description |
_PWM_MC_MDC_ENABLE |
Enable master duty cycle register. |
_PWM_MC_MDC_DISABLE |
Disable master duty cycle register. |
channel: PWM channel selection, valid values :
Value |
Description |
_PWM_MC_CH1 |
PWM channel 1. |
_PWM_MC_CH2 |
PWM channel 2. |
_PWM_MC_CH3 |
PWM channel 3. |
_PWM_MC_CH4 |
PWM channel 4. |
_PWM_MC_CH5 |
PWM channel 5. |
_PWM_MC_CH6 |
PWM channel 6. |
_PWM_MC_CH7 |
PWM channel 7. |
_PWM_MC_CH8 |
PWM channel 8. |
_PWM_MC_CH9 |
PWM channel 9. |
_PWM_MC_CH10 |
PWM channel 10. |
_PWM_MC_CH11 |
PWM channel 11. |
_PWM_MC_CH12 |
PWM channel 12. |
|
Returns |
Nothing.
|
Requires |
This routine should not be called after PWM is started.
|
Example |
PWM_MC_Init_Channel(_PWM_MC_ENABLE_LOW_PIN, _PWM_MC_TRUE_INDEPENDENT, _PWM_MC_CLK_PRIMARY, _PWM_MC_MDC_ENABLE, _PWM_MC_CH1);
|
Notes |
- This routine is avaliable only for dsPIC33EPXXX(GP/MC/MU)806/810/814 and PIC24EPXXX(GP/GU)810/814 family.
|
Library Example
The example changes PWM duty ratio on channel 1 continually.
If LED is connected to the channel 1, a gradual change of emitted light will be noticeable.
unsigned int i;
unsigned int duty_50;
void main(){
ADPCFG = 0xFFFF; // initialize AN pins as digital
PORTB = 0xAAAA;
TRISB = 0; // initialize portb as output
Delay_ms(1000);
duty_50 = PWM1_MC_Init(5000, 0, 0x01, 0); // Pwm_Mc_Init returns 50% of the duty
PWM1_MC_Set_Duty(i = duty_50, 1);
PWM1_MC_Start();
do
{
i--;
PWM1_MC_Set_Duty(i, 1);
Delay_ms(10);
if (i == 0)
i = duty_50 * 2 - 1; // Let us not allow the overflow
PORTB = i;
}
while(1);
}
HW Connection
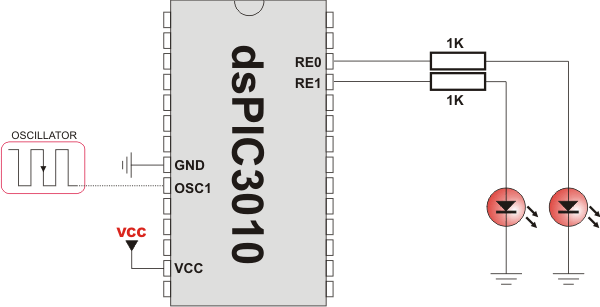
PWM Motor Control demonstration
Copyright (c) 2002-2018 mikroElektronika. All rights reserved.
What do you think about this topic ?
Send us feedback!
Want more examples and libraries?
Find them on