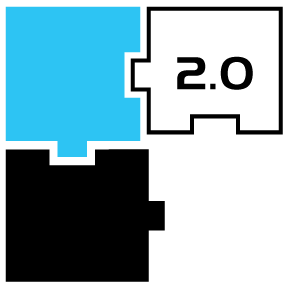 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
56 #define LCD_CMD_FUNCTION_SET ((uint8_t)0x20)
57 #define LCD_CMD_CLEAR ((uint8_t)0x1)
58 #define LCD_CMD_RETURN_HOME ((uint8_t)0x2)
59 #define LCD_CMD_CURSOR_OFF ((uint8_t)0xC)
60 #define LCD_CMD_UNDERLINE_ON ((uint8_t)0xE)
61 #define LCD_CMD_BLINK_CURSOR_ON ((uint8_t)0xF)
62 #define LCD_CMD_MOVE_CURSOR_LEFT ((uint8_t)0x10)
63 #define LCD_CMD_MOVE_CURSOR_RIGHT ((uint8_t)0x14)
64 #define LCD_CMD_TURN_OFF ((uint8_t)0x8)
65 #define LCD_CMD_TURN_ON ((uint8_t)0xC)
66 #define LCD_CMD_SHIFT_LEFT ((uint8_t)0x18)
67 #define LCD_CMD_SHIFT_RIGHT ((uint8_t)0x1C)
68 #define LCD_CMD_MODE_4BIT ((uint8_t)0x08)
74 #define LCD_MAP_PINS(lcd_cfg) LCD_MAP_PINS_4BIT(lcd_cfg)
79 #define LCD_MAP_PINS_4BIT(lcd_cfg) \
80 lcd_cfg.pin_cs = TFT_CS; \
81 lcd_cfg.pin_rst = TFT_RST; \
82 lcd_cfg.pin_backlight = TFT_BPWM; \
83 lcd_cfg.pin_d4 = TFT_D4; \
84 lcd_cfg.pin_d5 = TFT_D5; \
85 lcd_cfg.pin_d6 = TFT_D6; \
86 lcd_cfg.pin_d7 = TFT_D7;
91 #define LCD_MAP_PINS_8BIT(lcd_cfg) \
92 lcd_cfg.pin_cs = TFT_CS; \
93 lcd_cfg.pin_rst = TFT_RST; \
94 lcd_cfg.pin_backlight = TFT_BPWM; \
95 lcd_cfg.pin_d0 = TFT_D0; \
96 lcd_cfg.pin_d1 = TFT_D1; \
97 lcd_cfg.pin_d2 = TFT_D2; \
98 lcd_cfg.pin_d3 = TFT_D3; \
99 lcd_cfg.pin_d4 = TFT_D4; \
100 lcd_cfg.pin_d5 = TFT_D5; \
101 lcd_cfg.pin_d6 = TFT_D6; \
102 lcd_cfg.pin_d7 = TFT_D7;
init_sequence_ptr init_sequence
Controller init sequence.
Definition: lcd.h:202
void lcd_configure_default(lcd_config_t *config)
Configure LCD configuration structure.
struct lcd_handle lcd_handle_t
uint16_t waitBetweenWrites
Time to wait before generating a pulse on CS line.
Definition: lcd.h:177
void lcd_cursor_off(lcd_handle_t lcd_handle)
Removes cursor.
pin_name_t pin_d0
LCD data pins.
Definition: lcd.h:156
void lcd_clear(lcd_handle_t lcd_handle)
Clears LCD.
pin_name_t pin_cs
LCD control pins.
Definition: lcd.h:149
digital_out_t backlight_pin
Definition: lcd.h:195
void lcd_turn_on(lcd_handle_t lcd_handle)
Turns LCD ON.
void lcd_shift_left(lcd_handle_t lcd_handle)
Shifts data currently on LCD.
Digital output driver context structure, consisted of the following fields :
Definition: drv_digital_out.h:71
void lcd_write_text(lcd_handle_t lcd_handle, char *lcd_data, lcd_row_t row)
Writes text to LCD.
lcd_row_t
Definition: lcd.h:133
lcd_select_t
Definition: lcd.h:115
void lcd_backlight_on(lcd_handle_t lcd_handle)
Turns LCD backlight ON.
void lcd_shift_right(lcd_handle_t lcd_handle)
Shifts data currently on LCD.
digital_out_t rst_pin
Definition: lcd.h:194
pin_name_t pin_rst
Definition: lcd.h:150
digital_out_t data_pins[8]
Definition: lcd.h:196
pin_name_t pin_d1
Definition: lcd.h:157
void lcd_backlight_off(lcd_handle_t lcd_handle)
Turns LCD backlight OFF.
struct lcd_config lcd_config_t
mikroSDK supported LCD controller list.
lcd_config_t config
Definition: lcd.h:191
void lcd_cursor_move_home(lcd_handle_t lcd_handle)
Moves cursor to first position.
pin_name_t pin_d4
Definition: lcd.h:160
pin_name_t pin_d3
Definition: lcd.h:159
digital_out_t cs_pin
Definition: lcd.h:193
void lcd_write(lcd_handle_t lcd_handle, char lcd_cmd_data, lcd_select_t cmd_or_data)
Writes data to LCD.
pin_name_t pin_backlight
Definition: lcd.h:151
void lcd_set_row(lcd_handle_t lcd_handle, lcd_row_t row)
Sets current active row on LCD.
pin_name_t pin_d7
Definition: lcd.h:163
void lcd_cursor_on(lcd_handle_t lcd_handle)
Shows cursor.
pin_name_t pin_d5
Definition: lcd.h:161
lcd_err_t
Definition: lcd.h:106
pin_name_t pin_d6
Definition: lcd.h:162
hal_pin_name_t pin_name_t
Definition: drv_name.h:73
lcd_mode_t
Definition: lcd.h:124
bool init_state
Init state.
Definition: lcd.h:208
void lcd_cursor_move_right(lcd_handle_t lcd_handle)
Moves cursor position.
void(* init_sequence_ptr)(uint32_t)
Init sequence prototype.
Definition: lcd.h:184
void lcd_cursor_move_left(lcd_handle_t lcd_handle)
Moves cursor position.
pin_name_t pin_d2
Definition: lcd.h:158
void lcd_turn_off(lcd_handle_t lcd_handle)
Turns LCD OFF.
void lcd_init(lcd_handle_t lcd_handle, init_sequence_ptr init_sequence)
Initializes LCD.
lcd_err_t lcd_configure(lcd_handle_t *lcd_handle, lcd_config_t *config)
Configures LCD handle with passed configuration structure.
lcd_mode_t mode
LCD mode.
Definition: lcd.h:169