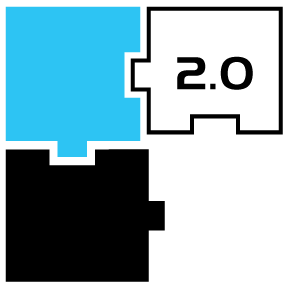 |
mikroSDK Reference Manual
|
Generic liquid crystal display APIs.
More...
|
| LCD Controller |
| Generic liquid crystal display controller specific APIs.
|
|
◆ lcd_configure_default()
Configures LCD structure to default initialization values. Take into consideration that this is just structure variable initial values setting. Values need to be redefined by user.
- Parameters
-
[in,out] | config | LCD driver configuration structure. See lcd_config_t structure definition for detailed explanation. |
Default values:
Function | Default value |
PIN_CS | HAL_PIN_NC |
PIN_RST | HAL_PIN_NC |
PIN_BACKLIGHT | HAL_PIN_NC |
PIN_D0 | HAL_PIN_NC |
PIN_D1 | HAL_PIN_NC |
PIN_D2 | HAL_PIN_NC |
PIN_D3 | HAL_PIN_NC |
PIN_D4 | HAL_PIN_NC |
PIN_D5 | HAL_PIN_NC |
PIN_D6 | HAL_PIN_NC |
PIN_D7 | HAL_PIN_NC |
MODE | LCD_MODE_BIT_4 |
WAITBETWEENWRITES | 1000 |
- Returns
- Nothing.
Example
- Note
- PIN_BACKLIGHT is not required.
◆ lcd_configure()
Configures LCD handle to values set in passed configuration structure. Additionaly will initialize all provided pins.
- Parameters
-
[in,out] | lcd_handle | LCD handle. See lcd_handle_t structure definition for detailed explanation. |
[in] | config | LCD driver configuration structure. See lcd_config_t structure definition for detailed explanation. |
- Returns
- lcd_err_t. Will return error if some of the pins weren't initialized correctly.
Example
◆ lcd_init()
Initializes LCD with default values.
- Parameters
-
[in] | lcd_handle | LCD handle. |
[in] | init_sequence_ptr | Pointer to init sequence API. See lcd_handle_t structure definition for detailed explanation. |
- Returns
- Nothing.
Example
- Note
- init_sequence_ptr can be declared by user as well.
◆ lcd_write()
Writes either commands or data to LCD lines.
- Parameters
-
[in] | lcd_handle | LCD handle. See lcd_handle_t structure definition for detailed explanation. |
[in] | lcd_cmd_data | Data or command. |
[in] | cmd_or_data | Selector for pulse signaling. See lcd_select_t enum definition for detailed explanation. |
- Returns
- Nothing.
Example
◆ lcd_write_text()
Writes array of characters to LCD lines one by one.
- Parameters
-
[in] | lcd_handle | LCD handle. See lcd_handle_t structure definition for detailed explanation. |
[in] | lcd_data | Data. |
[in] | row | Selects row to write to. See lcd_row_t enum definition for detailed explanation. |
- Returns
- Nothing.
Example
◆ lcd_shift_right()
Shifts the data on all rows on LCD one character at a time.
- Parameters
-
- Returns
- Nothing.
Example
for(char i=0; i<8; i++) {
}
◆ lcd_shift_left()
Shifts the data on all rows on LCD one character at a time.
- Parameters
-
- Returns
- Nothing.
Example
for(char i=0; i<8; i++) {
}
◆ lcd_set_row()
Sets active row on the LCD where data is to be output.
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_turn_on()
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_turn_off()
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_clear()
Clears all characters on LCD.
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_cursor_move_home()
Moves current cursor position back to the home position.
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_cursor_move_left()
Moves current cursor position one column to the left.
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_cursor_move_right()
Moves current cursor position one column to the right.
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_cursor_off()
Removes cursor from current position on the display.
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_cursor_on()
Shows current cursor position on the display.
- Parameters
-
- Returns
- Nothing.
Example
- Attention
- Turns cursor blinking ON for better visibility as well.
◆ lcd_backlight_off()
- Parameters
-
- Returns
- Nothing.
Example
◆ lcd_backlight_on()
- Parameters
-
- Returns
- Nothing.
Example
void lcd_configure_default(lcd_config_t *config)
Configure LCD configuration structure.
void lcd_cursor_off(lcd_handle_t lcd_handle)
Removes cursor.
void hd44780_lcd_init(uint32_t lcd_handle)
Initializes LCD.
void lcd_clear(lcd_handle_t lcd_handle)
Clears LCD.
void lcd_turn_on(lcd_handle_t lcd_handle)
Turns LCD ON.
void lcd_shift_left(lcd_handle_t lcd_handle)
Shifts data currently on LCD.
void lcd_write_text(lcd_handle_t lcd_handle, char *lcd_data, lcd_row_t row)
Writes text to LCD.
void lcd_backlight_on(lcd_handle_t lcd_handle)
Turns LCD backlight ON.
void lcd_shift_right(lcd_handle_t lcd_handle)
Shifts data currently on LCD.
void lcd_backlight_off(lcd_handle_t lcd_handle)
Turns LCD backlight OFF.
#define LCD_MAP_PINS(lcd_cfg)
Mapping default LCD control and data pins.
Definition: lcd.h:73
void lcd_cursor_move_home(lcd_handle_t lcd_handle)
Moves cursor to first position.
void lcd_write(lcd_handle_t lcd_handle, char lcd_cmd_data, lcd_select_t cmd_or_data)
Writes data to LCD.
void Delay_ms(uint32_t time_ms)
Creates a software delay in duration of time_ms milliseconds.
void lcd_set_row(lcd_handle_t lcd_handle, lcd_row_t row)
Sets current active row on LCD.
void lcd_cursor_on(lcd_handle_t lcd_handle)
Shows cursor.
void lcd_cursor_move_right(lcd_handle_t lcd_handle)
Moves cursor position.
void lcd_cursor_move_left(lcd_handle_t lcd_handle)
Moves cursor position.
void lcd_turn_off(lcd_handle_t lcd_handle)
Turns LCD OFF.
void lcd_init(lcd_handle_t lcd_handle, init_sequence_ptr init_sequence)
Initializes LCD.
lcd_err_t lcd_configure(lcd_handle_t *lcd_handle, lcd_config_t *config)
Configures LCD handle with passed configuration structure.