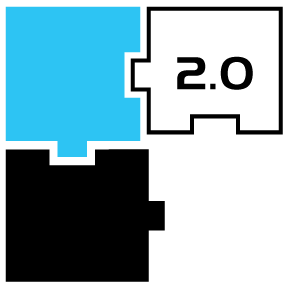 |
mikroSDK Reference Manual
|
UART Driver API Reference.
More...
|
void | uart_configure_default (uart_config_t *config) |
| Configure UART Driver configuration structure. More...
|
|
err_t | uart_open (uart_t *obj, uart_config_t *config) |
| Open the UART Driver object. More...
|
|
err_t | uart_set_baud (uart_t *obj, uint32_t baud) |
| Set the UART baud rate. More...
|
|
err_t | uart_set_parity (uart_t *obj, uart_parity_t parity) |
| Set the UART parity. More...
|
|
err_t | uart_set_stop_bits (uart_t *obj, uart_stop_bits_t stop) |
| Set the number of UART stop bits. More...
|
|
err_t | uart_set_data_bits (uart_t *obj, uart_data_bits_t bits) |
| Set the number of UART data bits. More...
|
|
void | uart_set_blocking (uart_t *obj, bool blocking) |
| Set UART Driver in blocking/non-blocking mode. More...
|
|
err_t | uart_write (uart_t *obj, uint8_t *buffer, size_t size) |
| Write data to UART. More...
|
|
err_t | uart_print (uart_t *obj, char *text) |
| Print the string to UART. More...
|
|
err_t | uart_println (uart_t *obj, char *text) |
| Print the string to UART and append new line. More...
|
|
err_t | uart_read (uart_t *obj, uint8_t *buffer, size_t size) |
| Read data from UART. More...
|
|
size_t | uart_bytes_available (uart_t *obj) |
| Check number of bytes available to read from UART. More...
|
|
void | uart_clear (uart_t *obj) |
| Discard all characters from UART buffers. More...
|
|
err_t | uart_close (uart_t *obj) |
| Close UART Driver object. More...
|
|
UART DRV API layer header. Contains all enums, structures and function declarations available for UART module.
◆ uart_configure_default()
Configures uart_config_t structure to default initialization values. Take into consideration that this is just structure variable initial values setting. Values need to be redefined by user.
- Parameters
-
[in,out] | config | configure UART driver configuration structure. See uart_config_t structure definition for detailed explanation. |
Default values:
Function | Default value |
Tx pin | 0xFFFFFFFF (invalid pin) |
Rx pin | 0xFFFFFFFF (invalid pin) |
Baud rate | 115200 |
Data bits | 8 data bits |
Parity | no parity |
Stop bits | 1 stop bit |
Tx buffer | cleared |
Rx buffer | cleared |
Tx ring buffer size | zero |
Rx ring buffer size | zero |
- Returns
- Nothing.
Example
◆ uart_open()
Opens the UART driver object on selected pins. Allocates memory, pins and ring buffers for specified object. Also, initializes interrupts on the global level.
- Parameters
-
[in,out] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | config | configure UART driver configuration settings. See uart_config_t structure definition for detailed explanation. |
- Returns
- The function can return one of the values defined by err_t, which is dependant on the architecture and ported low level layer.
- Precondition
- Make sure that
configuration
structure has been adequately populated beforehand. See uart_configure_default definition for detailed explanation.
- Note
- It is recommended to check return value for error.
Example
static uint8_t uart_rx_buffer[128];
static uint8_t uart_tx_buffer[128];
uart_cfg.
rx_pin = MIKROBUS_1_RX;
uart_cfg.
tx_pin = MIKROBUS_1_TX;
{
}
◆ uart_set_baud()
Sets the baud rate to specified baud
value.
- Parameters
-
[in] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | baud | Specified baud rate value. |
- Returns
- The function can return one of the values defined by err_t, which is dependant on the architecture and ported low level layer.
- Precondition
- Make sure that adequate memory has been allocated beforehand. See uart_open definition for detailed explanation.
- Note
- It is recommended to check return value for error.
Example
◆ uart_set_parity()
Sets parity scheme to be used by the UART driver.
- Parameters
-
[in,out] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | parity | Parity. See uart_parity_t for valid values. |
- Returns
- The function can return one of the values defined by err_t, which is dependant on the architecture and ported low level layer.
- Precondition
- Make sure that adequate memory has been allocated beforehand. See uart_open definition for detailed explanation.
- Note
- It is recommended to check return value for error.
Example
◆ uart_set_stop_bits()
Sets the number of stop bits to be used by the UART driver.
- Parameters
-
[in,out] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | stop | Stop bits. See uart_stop_bits_t for valid values. |
- Returns
- The function can return one of the values defined by err_t, which is dependant on the architecture and ported low level layer.
- Precondition
- Make sure that adequate memory has been allocated beforehand. See uart_open definition for detailed explanation.
- Note
- It is recommended to check return value for error.
Example
◆ uart_set_data_bits()
Sets the number of data bits to be used by the UART driver.
- Parameters
-
[in,out] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | bits | Data bits. See uart_data_bits_t for valid values. |
- Returns
- The function can return one of the values defined by err_t, which is dependant on the architecture and ported low level layer.
- Precondition
- Make sure that adequate memory has been allocated beforehand. See uart_open definition for detailed explanation.
- Note
- It is recommended to check return value for error.
Example
◆ uart_set_blocking()
void uart_set_blocking |
( |
uart_t * |
obj, |
|
|
bool |
blocking |
|
) |
| |
Sets the UART driver to work in blocking/non-blocking mode.
- Parameters
-
[in,out] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | blocking | true for blocking mode, false for non-blocking mode. |
- Returns
- Nothing.
Example
◆ uart_write()
err_t uart_write |
( |
uart_t * |
obj, |
|
|
uint8_t * |
buffer, |
|
|
size_t |
size |
|
) |
| |
Writes at most size
bytes of data from buffer
to the device.
- Parameters
-
[in] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | buffer | Array containing data to be written. |
[in] | size | Number of bytes to be written. |
- Returns
- Returns the number of bytes that were actually written, or -1 if an error occurred.
- Precondition
- Make sure that adequate memory has been allocated beforehand, and the module was configured adequately ( bit-rate... ). See uart_open, uart_set_baud, uart_set_data_bits, uart_set_stop_bits and uart_set_parity definition for detailed explanation.
- Note
- Take into consideration that the function shall return (-1) if no data was written, and appropriate error handling strategy is recommended.
Example
uint8_t *uart_tx_buffer;
static size_t size;
◆ uart_print()
Print the string pointed to by text.
- Parameters
-
[in] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | text | Pointer to text array. |
- Returns
- Returns the number of bytes that were actually written, or -1 if an error occurred.
- Precondition
- Make sure that adequate memory has been allocated beforehand, and the module was configured adequately ( bit-rate... ). See uart_open, uart_set_baud, uart_set_data_bits, uart_set_stop_bits and uart_set_parity definition for detailed explanation.
- Note
- Take into consideration that the function shall return (-1) if no data was written, and appropriate error handling strategy is recommended.
Example
◆ uart_println()
Print the string pointed to by text and append new line at the end.
- Parameters
-
[in] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[in] | text | Pointer to text array. |
- Returns
- Returns the number of bytes that were actually written, or -1 if an error occurred.
- Precondition
- Make sure that adequate memory has been allocated beforehand, and the module was configured adequately ( bit-rate... ). See uart_open, uart_set_baud, uart_set_data_bits, uart_set_stop_bits and uart_set_parity definition for detailed explanation.
- Note
- Take into consideration that the function shall return (-1) if no data was written, and appropriate error handling strategy is recommended.
Example
static size_t size;
size = uart_print_ln( &uart, "Hello!" );
◆ uart_read()
err_t uart_read |
( |
uart_t * |
obj, |
|
|
uint8_t * |
buffer, |
|
|
size_t |
size |
|
) |
| |
Reads at most size
bytes of data from the device into buffer
.
- Parameters
-
[in] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
[out] | buffer | Array to place read data in. |
[in] | size | Number of bytes to be written. |
- Returns
- Returns the number of bytes that were actually read, or -1 if an error occurred.
- Precondition
- Make sure that adequate memory has been allocated beforehand, and the module was configured adequately ( bit-rate... ). See uart_open, uart_set_baud, uart_set_data_bits, uart_set_stop_bits and uart_set_parity definition for detailed explanation.
- Note
- Take into consideration that the function shall return (-1) if no data was read, and appropriate error handling strategy is recommended.
Example
uint8_t *uart_rx_buffer;
static size_t size;
size =
uart_read( &uart, uart_rx_buffer,
sizeof( buffer ) );
◆ uart_bytes_available()
size_t uart_bytes_available |
( |
uart_t * |
obj | ) |
|
Check number of bytes available to read from UART.
- Parameters
-
[in] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
- Returns
- Returns the number of bytes that are available for reading.
Example
◆ uart_clear()
void uart_clear |
( |
uart_t * |
obj | ) |
|
Discards all characters from the output and input buffer.
- Parameters
-
[in] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
- Returns
- Nothing.
Example
◆ uart_close()
Closes UART driver object, disables all interrupts, resets pin AF to default values, clears all buffers used by object and disables module clock for lower power consumption.
- Parameters
-
[in,out] | obj | UART driver object. See uart_t structure definition for detailed explanation. |
- Returns
- The function can return one of the values defined by err_t, which is dependant on the architecture and ported low level layer.
Example
Definition: drv_uart.h:60
void uart_clear(uart_t *obj)
Discard all characters from UART buffers.
size_t rx_ring_size
Definition: drv_uart.h:150
void uart_set_blocking(uart_t *obj, bool blocking)
Set UART Driver in blocking/non-blocking mode.
err_t uart_print(uart_t *obj, char *text)
Print the string to UART.
size_t tx_ring_size
Definition: drv_uart.h:149
Definition: drv_uart.h:84
err_t uart_open(uart_t *obj, uart_config_t *config)
Open the UART Driver object.
Definition: drv_uart.h:97
err_t uart_close(uart_t *obj)
Close UART Driver object.
err_t uart_set_stop_bits(uart_t *obj, uart_stop_bits_t stop)
Set the number of UART stop bits.
err_t uart_set_parity(uart_t *obj, uart_parity_t parity)
Set the UART parity.
UART driver context structure, consisted of the following fields :
Definition: drv_uart.h:163
Definition: drv_uart.h:59
Definition: drv_uart.h:72
err_t uart_set_data_bits(uart_t *obj, uart_data_bits_t bits)
Set the number of UART data bits.
pin_name_t rx_pin
Definition: drv_uart.h:139
err_t uart_write(uart_t *obj, uint8_t *buffer, size_t size)
Write data to UART.
err_t uart_read(uart_t *obj, uint8_t *buffer, size_t size)
Read data from UART.
size_t uart_bytes_available(uart_t *obj)
Check number of bytes available to read from UART.
UART init configuration structure, consisted of the following fields :
Definition: drv_uart.h:136
err_t uart_set_baud(uart_t *obj, uint32_t baud)
Set the UART baud rate.
pin_name_t tx_pin
Definition: drv_uart.h:138
void uart_configure_default(uart_config_t *config)
Configure UART Driver configuration structure.