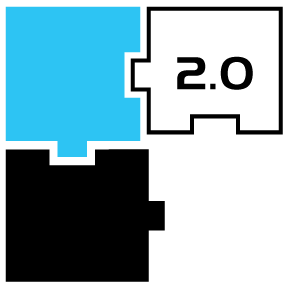 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
170 uint8_t *tx_ring_buffer;
171 uint8_t *rx_ring_buffer;
173 bool is_tx_irq_enabled;
174 bool is_rx_irq_enabled;
614 #endif // _DRV_UART_H_
Definition: drv_uart.h:60
Pin and port name type definitions.
void uart_clear(uart_t *obj)
Discard all characters from UART buffers.
Definition: drv_uart.h:81
Definition: drv_uart.h:82
void uart_set_blocking(uart_t *obj, bool blocking)
Set UART Driver in blocking/non-blocking mode.
err_t uart_print(uart_t *obj, char *text)
Print the string to UART.
uart_data_bits_t
Definition: drv_uart.h:66
Definition: drv_uart.h:84
uart_parity_t
Definition: drv_uart.h:78
err_t uart_open(uart_t *obj, uart_config_t *config)
Open the UART Driver object.
Definition: drv_uart.h:69
uart_stop_bits_t
Definition: drv_uart.h:90
err_t uart_println(uart_t *obj, char *text)
Print the string to UART and append new line.
Definition: drv_uart.h:97
err_t uart_close(uart_t *obj)
Close UART Driver object.
Definition: drv_uart.h:94
uart_err_t
Definition: drv_uart.h:57
err_t uart_set_stop_bits(uart_t *obj, uart_stop_bits_t stop)
Set the number of UART stop bits.
err_t uart_set_parity(uart_t *obj, uart_parity_t parity)
Set the UART parity.
UART driver context structure, consisted of the following fields :
Definition: drv_uart.h:163
Definition: drv_uart.h:59
Definition: drv_uart.h:72
err_t uart_set_data_bits(uart_t *obj, uart_data_bits_t bits)
Set the number of UART data bits.
err_t uart_write(uart_t *obj, uint8_t *buffer, size_t size)
Write data to UART.
Definition: drv_uart.h:95
Definition: drv_uart.h:80
err_t uart_read(uart_t *obj, uint8_t *buffer, size_t size)
Read data from UART.
Definition: drv_uart.h:70
Definition: drv_uart.h:68
size_t uart_bytes_available(uart_t *obj)
Check number of bytes available to read from UART.
UART init configuration structure, consisted of the following fields :
Definition: drv_uart.h:136
err_t uart_set_baud(uart_t *obj, uint32_t baud)
Set the UART baud rate.
hal_pin_name_t pin_name_t
Definition: drv_name.h:73
int32_t err_t
Definition: hal_target.h:63
void uart_configure_default(uart_config_t *config)
Configure UART Driver configuration structure.
Definition: drv_uart.h:92
Definition: drv_uart.h:93