ADC Library
ADC (Analog to Digital Converter) module is available with a number of dsPIC30/33 and PIC24 MCU modules. ADC is an electronic circuit that converts continuous signals to discrete digital numbers. ADC Library provides you a comfortable work with the module.
Library Routines
ADCx_Init
Prototype |
void ADCx_Init();
|
Description |
This routines configures ADC module to work with default settings.
The internal ADC module is set to:
- single channel conversion
- 10-bit conversion resolution
- unsigned integer data format
- auto-convert
- VRef+ : AVdd, VRef- : AVss
- instruction cycle clock
- conversion clock : 32*Tcy
- auto-sample time : 31TAD
|
Parameters |
None.
|
Returns |
Nothing.
|
Requires |
- MCU with built-in ADC module.
- ADC library routines require you to specify the module you want to use. To select the desired ADC module, simply change the letter x in the routine prototype for a number from 1 to 2.
|
Example |
ADC1_Init(); // Initialize ADC1 module with default settings
|
Notes |
- Number of ADC modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
|
ADCx_Init_Advanced
Prototype |
// dsPIC30F and PIC24FJ prototype
void ADC1_Init_Advanced(unsigned Reference);
// dsPIC33FJ and PIC24HJ prototype
void ADCx_Init_Advanced(unsigned ADCMode, unsigned Reference);
// dsPIC33EVXXXGM00X_10X
void ADCx_Init_Advanced(unsigned ADCMode, unsigned channel);
|
Description |
This routine configures the internal ADC module to work with user defined settings.
|
Parameters |
ADCMode: resolution of the ADC module.
Reference: voltage reference used in ADC process.
channel represents the channel from which the analog value is to be acquired (only for dsPIC33EVXXXGM00X_10X).
Description |
Predefined library const |
ADC mode: |
10-bit resolution |
_ADC_10bit |
12-bit resolution |
_ADC_12bit |
Voltage reference: |
Internal voltage reference (from AVdd and AVss) |
_ADC_INTERNAL_REF |
External voltage reference (from VREF+ and VREF- Pins) |
_ADC_EXTERNAL_VREF |
Internal Positive Voltage Reference from AVdd |
_ADC_INTERNAL_VREFH |
Internal Negative Voltage Reference from AVss |
_ADC_INTERNAL_VREFL |
External Positive Voltage Reference from VREF+ Pin |
_ADC_EXTERNAL_VREFH |
External Negative Voltage Reference from VREF- Pin |
_ADC_EXTERNAL_VREFL |
Internal Positive Voltage Reference VRH1 (2 * Internal VBG) |
_ADC_INTERNAL_VRH1 |
Internal Positive Voltage Reference VRH2 (4 * Internal VBG) |
_ADC_INTERNAL_VRH2 |
|
Returns |
Nothing.
|
Requires |
- The MCU with built-in ADC module.
- ADC library routines require you to specify the module you want to use. To select the desired ADC module, simply change the letter x in the routine prototype for a number from 1 to 2.
|
Example |
ADC1_Init_Advanced(_ADC_10bit, _ADC_INTERNAL_REF); // sets ADC module in 10-bit resolution mode with internal reference used
ADC1_Init_Advanced(_ADC_12bit, _ADC_INTERNAL_VREFL | _ADC_INTERNAL_VREFH); // sets ADC module in 12-bit resolution mode with the following reference : AVss and VREF+
|
Notes |
- Number of ADC modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
- Not all MCUs support advanced configuration. Please, read the appropriate datasheet before utilizing this library.
|
ADCx_Get_Sample
Prototype |
unsigned ADCx_Get_Sample(unsigned channel);
|
Description |
The function enables ADC module and reads the specified analog channel input.
|
Parameters |
channel represents the channel from which the analog value is to be acquired.
|
Returns |
10-bit or 12-bit (depending on selected mode by ADCx_Init_Advanced or MCU) unsigned value from the specified channel .
|
Requires |
- The MCU with built-in ADC module.
- Prior to using this routine, ADC module needs to be initialized. See ADCx_Init and ADCx_Init_Advanced.
- ADC library routines require you to specify the module you want to use. To select the desired ADC module, simply change the letter x in the routine prototype for a number from 1 to 2.
- Before using the function, be sure to configure the appropriate TRISx bits to designate pins as inputs.
|
Example |
unsigned adc_value;
...
adc_value = ADC1_Get_Sample(10); // read analog value from ADC1 module channel 10
|
Notes |
- Number of ADC modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
- The function sets the appropriate bit in the ADPCFG registers to enable analog function of the chosen pin.
- Refer to the appropriate Datasheet for channel-to-pin mapping.
|
ADCx_Read
Prototype |
unsigned ADCx_Read(unsigned channel);
|
Description |
The function initializes, enables ADC module and reads the specified analog channel input.
|
Parameters |
channel represents the channel from which the analog value is to be acquired.
|
Returns |
10-bit or 12-bit (depending on the MCU) unsigned value from the specified channel .
|
Requires |
- The MCU with built-in ADC module.
- ADC library routines require you to specify the module you want to use. To select the desired ADC module, simply change the letter x in the routine prototype for a number from 1 to 2.
- Number of ADC modules per MCU differs from chip to chip. Please, read the appropriate datasheet before utilizing this library.
- Before using the function, be sure to configure the appropriate TRISx bits to designate pins as inputs.
|
Example |
unsigned adc_value;
...
adc_value = ADC1_Read(10); // read analog value from ADC1 module channel 10
|
Notes |
- This is a standalone routine, so there is no need for a previous initialization of ADC module.
- The function sets the appropriate bit in the ADPCFG registers to enable analog function of the chosen pin.
- Refer to the appropriate Datasheet for channel-to-pin mapping.
|
ADC_Set_Active
Prototype |
void ADC_Set_Active(unsigned (*adc_gs)(unsigned));
|
Description |
Sets active ADC module.
|
Parameters |
Parameters :
|
Returns |
Nothing.
|
Requires |
Routine is available only for MCUs with multiple ADC modules.
Used ADC module must be initialized before using this routine. See ADCx_Init and ADCx_Init_Advanced routines.
|
Example |
// Activate ADC2 module
ADC_Set_Active(ADC2_Get_Sample);
|
Notes |
None.
|
ADC1_ActivateCore
Prototype |
void ADC1_ActivateCore(unsigned ADCCore);
|
Description |
Sets used ADC conversion core.
|
Parameters |
ADCCore: conversion core. Valid values :
Value |
Description |
_ADC_SHCORE |
ADC Shared Core |
_ADC_CORE0 |
ADC Core 0 |
_ADC_CORE1 |
ADC Core 1 |
_ADC_CORE2 |
ADC Core 2 |
_ADC_CORE3 |
ADC Core 3 |
|
Returns |
Nothing.
|
Requires |
- Routine is available only for dsPIC33EPXXGS50X an dsPIC33EPXXGS202 MCUs.
- Used ADC module must be initialized before using this routine. See ADCx_Init and ADCx_Init_Advanced routines.
|
Example |
|
Notes |
- This function must be called before ADCx_Get_Sample and ADCx_Read functions are used.
- Refer to the datasheet for the ADC channel to ADC core mapping.
|
Library Example
This code snippet reads analog value from the channel 1 and sends readings as a text over UART1.
unsigned adcRes;
char txt[6];
void main() {
PORTB = 0x0000;
TRISB.F1 = 1; // set pin as input - needed for ADC to work
ADC1_Init();
UART1_Init(9600);
while (1) {
adcRes = ADC1_Get_Sample(1);
WordToStr(adcRes, txt);
UART1_Write_Text(txt);
Delay_ms(50);
}
}
HW Connection
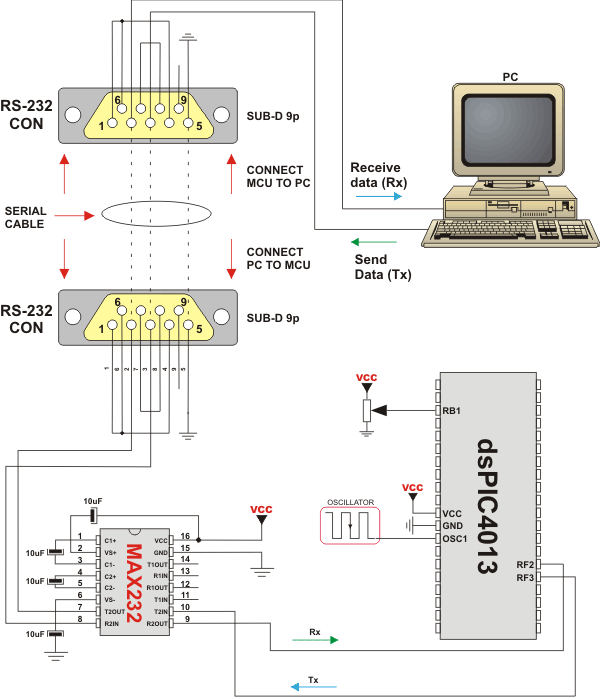
ADC HW connection
Copyright (c) 2002-2018 mikroElektronika. All rights reserved.
What do you think about this topic ?
Send us feedback!
Want more examples and libraries?
Find them on