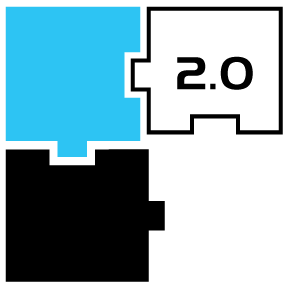 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
43 #ifndef _VTFT_TYPES_H_
44 #define _VTFT_TYPES_H_
47 #include "generic_pointer.h"
613 uint32_t min_position;
614 uint32_t max_position;
670 #endif // _VTFT_TYPES_H_
Structure representig a screen.
Definition: vtft_types.h:629
Touch Panel Context Object.
Definition: tp.h:222
Definition: vtft_types.h:66
Definition: vtft_types.h:121
The structure used for information about the gradient displayed when an object is pressed and when it...
Definition: vtft_types.h:149
struct vtft_s vtft_t
Structure representig a VTFT library instance.
uint8_t vtft_byte_t
Definition: vtft_types.h:51
vtft_bool_t pen_down
Definition: vtft_types.h:661
Structure for a componet drawn like a line.
Definition: vtft_types.h:378
Structure for an image component.
Definition: vtft_types.h:496
vtft_active_component *__generic current_active_component
Definition: vtft_types.h:664
Definition: vtft_types.h:120
vtft_text_alignment
Enum containing possible text alignment options.
Definition: vtft_types.h:199
Structure representig a VTFT library instance.
Definition: vtft_types.h:654
Declaration of types for Graphic Library.
Definition: vtft_types.h:71
vtft_component_type
Enum containing all VTFT component types.
Definition: vtft_types.h:60
Definition: vtft_types.h:62
vtft_component_type vtft_comp_type_t
Definition: vtft_types.h:80
gl_int_t vtft_coord_t
Definition: vtft_types.h:53
Definition: vtft_types.h:64
void(* vtft_draw_handle)(struct vtft_s *instance, vtft_component *__generic component)
Definition: vtft_types.h:645
Structure representing an event set, all posible event types and their functions that can be triggere...
Definition: vtft_types.h:92
The structure used for information about the gradient.
Definition: vtft_types.h:133
The context structure for storing pen's size and color.
Definition: vtft_types.h:107
void(* vtft_event)()
Definition: vtft_types.h:84
Definition: vtft_types.h:76
vtft_gradient_style
Definition: vtft_types.h:117
gl_uint_t vtft_ucoord_t
Definition: vtft_types.h:54
Structure for a label component.
Definition: vtft_types.h:399
Generic component structure, holding key information about that component.
Definition: vtft_types.h:218
The structure used for information about the font bitmap and color.
Definition: vtft_types.h:187
Definition: vtft_types.h:63
Structure for a componet drawn like a ellipse.
Definition: vtft_types.h:356
Structure for a radio button component.
Definition: vtft_types.h:595
Definition: vtft_types.h:70
uint16_t gl_color_t
Definition: gl_colors.h:55
Abstract structure for check box component.
Definition: vtft_types.h:518
Definition: vtft_types.h:205
The structure used for information about the font bitmap and color.
Definition: vtft_types.h:168
int16_t gl_int_t
Definition: gl_types.h:90
Structure for a componet drawn like a circle.
Definition: vtft_types.h:335
Structure for a check box component.
Definition: vtft_types.h:543
Structure for a componet characterized by position.
Definition: vtft_types.h:233
Definition: vtft_types.h:122
Definition: vtft_types.h:206
Definition: vtft_types.h:123
Definition: vtft_types.h:68
uint8_t vtft_bool_t
Definition: vtft_types.h:50
uint16_t gl_uint_t
Definition: gl_types.h:91
int16_t vtft_index_t
Definition: vtft_types.h:52
Definition: vtft_types.h:74
Definition: vtft_types.h:203
vtft_screen * current_screen
Definition: vtft_types.h:659
vtft_bool_t screen_changed
Definition: vtft_types.h:662
Structure for a componet drawn like a box.
Definition: vtft_types.h:290
Definition: vtft_types.h:73
Definition: vtft_types.h:69
vtft_active_component *__generic pressed_component
Definition: vtft_types.h:665
Structure for a componet characterized by color used for drawing it.
Definition: vtft_types.h:268
Definition: vtft_types.h:72
Definition: vtft_types.h:67
Structure for a componet characterized by active and inactive state.
Definition: vtft_types.h:250
Definition: vtft_types.h:204
Definition: vtft_types.h:75
Definition: vtft_types.h:119
Definition: vtft_types.h:202
tp_t * tp_instance
Definition: vtft_types.h:656
Structure for a componet drawn like a box with rounded corners.
Definition: vtft_types.h:312
Definition: vtft_types.h:65
Definition: vtft_types.h:201
vtft_draw_handle draw_handles[VTFT_COMPONENT_COUNT]
Definition: vtft_types.h:658