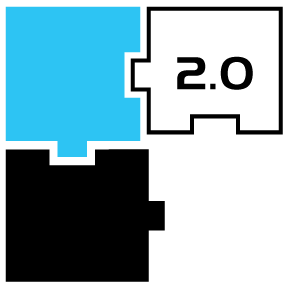 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
75 #define configureData(c,pd,m) ((c<<4)|(pd<<2)|(m<<1))
146 uint16_t tsc2003_pressure_threshold_lower;
147 uint16_t tsc2003_pressure_threshold_upper;
232 #define TSC2003_I2C_SLAVE_ADDR_FIXED (0x48)
238 #define TSC2003_I2C_SLAVE_ADDR_A0 (0x1)
244 #define TSC2003_I2C_SLAVE_ADDR_A1 (0x2)
250 #define TSC2003_I2C_SLAVE_ADDR_0 (TSC2003_I2C_SLAVE_ADDR_FIXED)
256 #define TSC2003_I2C_SLAVE_ADDR_1 (TSC2003_I2C_SLAVE_ADDR_FIXED | \
257 TSC2003_I2C_SLAVE_ADDR_A0)
263 #define TSC2003_I2C_SLAVE_ADDR_2 (TSC2003_I2C_SLAVE_ADDR_FIXED | \
264 TSC2003_I2C_SLAVE_ADDR_A1)
270 #define TSC2003_I2C_SLAVE_ADDR_3 (TSC2003_I2C_SLAVE_ADDR_FIXED | \
271 TSC2003_I2C_SLAVE_ADDR_A0 | \
272 TSC2003_I2C_SLAVE_ADDR_A1)
278 #define TSC2003_I2C_SLAVE_ADDR_DEFAULT (TSC2003_I2C_SLAVE_ADDR_3)
290 #define TSC2003_MAP_PINS( cfg ) \
291 cfg.i2c_cfg.scl = CTP_SCL; \
292 cfg.i2c_cfg.sda = CTP_SDA; \
293 cfg.int_pin = CTP_INT
481 #endif // _TSC2003_H_
Pin and port name type definitions.
void tsc2003_press_coordinates(tsc2003_t *ctx, tp_touch_item_t *touch_item)
Copies touch information from context object to touch item object.
I2C Master driver context structure, consisted of the following fields :
Definition: drv_i2c_master.h:119
Definition: tsc2003.h:110
void tsc2003_set_pressure_threshold_level(tsc2003_cfg_t *cfg, tsc2003_pressure_threshold_t pressure)
Utility funciton used for setting threshold levels.
Definition: tsc2003.h:111
void tsc2003_gesture(tsc2003_t *ctx, tp_event_t *event)
Read gesture information.
void tsc2003_cfg_setup(tsc2003_cfg_t *cfg)
TSC2003 configuration object setup function.
void tsc2003_calibrate(tsc2003_t *ctx)
Calibrates variables used for converting raw TSC2003 ADC values to pixel coordinates.
API for Digital input driver.
tp_event_t
Touch Panel Event Code Definition.
Definition: tp.h:79
tp_event_t tsc2003_press_detect(tsc2003_t *ctx)
Press detection function.
void tsc2003_default_cfg(tsc2003_t *ctx)
TSC2003 Default Configuration Function.
Digital input driver context structure, consisted of the following fields :
Definition: drv_digital_in.h:71
Touch Item Definition.
Definition: tp.h:149
tp_err_t tsc2003_init(tsc2003_t *ctx, tsc2003_cfg_t *cfg, tp_drv_t *drv)
TSC2003 Initialization Function.
tp_err_t tsc2003_process(tsc2003_t *ctx)
Function processing events.
Definition: tsc2003.h:113
tsc2003_bits_m
TSC2003 Mode.
Definition: tsc2003.h:124
Press intensity needed to register touch event.
Definition: tsc2003.h:144
void tsc2003_get_calibration_data(tsc2003_t *ctx, tsc2003_calibration_data_t *cdata)
Utility funciton used for getting a copy of current calibration data.
void tsc2003_set_calibration_data(tsc2003_t *ctx, const tsc2003_calibration_data_t *cdata)
Utility funciton used for setting calibration data.
tsc2003_bits_cfg
TSC2003 Configuration.
Definition: tsc2003.h:85
tp_err_t
Touch Panel Error Code Definition.
Definition: tp.h:63
API for I2C master driver.
Calibration data structure.
Definition: tsc2003.h:133
Definition: tsc2003.h:112
hal_pin_name_t pin_name_t
Definition: drv_name.h:73
API for Digital output driver.
void tsc2003_generic_read(tsc2003_t *ctx, uint8_t reg_addr, uint8_t *data_buff, uint8_t len)
Reads a number of bytes from a register.
Definition: tsc2003.h:126
TSC2003 Context Object.
Definition: tsc2003.h:183
Touch Panel Driver Interface Items.
Definition: tp.h:197
void tsc2003_write_cmd(tsc2003_t *ctx, uint8_t reg_addr)
Writes a generic command to the IC.
TSC2003 Configuration Object.
Definition: tsc2003.h:160
tsc2003_bits_pd
TSC2003 Power Down Setting.
Definition: tsc2003.h:109
const tsc2003_calibration_data_t TSC2003_CALIBRATION_DATA_DEFAULT
Default calibration data specific for MikroE resistive tft boards.
I2C Master initialization configuration structure, consisted of the following fields :
Definition: drv_i2c_master.h:95
Definition: tsc2003.h:125