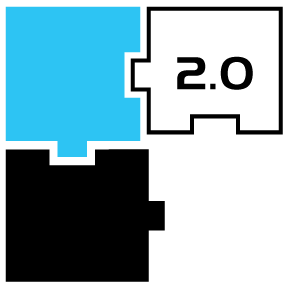 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
64 #define VREF_VALUE 3.3f
66 #ifndef TP_MIKROE_CALIBRATION_XMIN
71 #define TP_MIKROE_CALIBRATION_XMIN 0
73 #ifndef TP_MIKROE_CALIBRATION_XMAX
78 #define TP_MIKROE_CALIBRATION_XMAX 0
81 #ifndef TP_MIKROE_CALIBRATION_YMIN
86 #define TP_MIKROE_CALIBRATION_YMIN 0
88 #ifndef TP_MIKROE_CALIBRATION_YMAX
93 #define TP_MIKROE_CALIBRATION_YMAX 0
96 #ifndef TP_MIKROE_PRESSURE_THRESHOLD_LOWER
101 #define TP_MIKROE_PRESSURE_THRESHOLD_LOWER 0
103 #ifndef TP_MIKROE_PRESSURE_THRESHOLD_UPPER
108 #define TP_MIKROE_PRESSURE_THRESHOLD_UPPER 0
114 #define TP_MIKROE_MAP_PINS( tp_mikroe_cfg ) \
115 tp_mikroe_cfg.left = TP_MIKROE_XL;\
116 tp_mikroe_cfg.right = TP_MIKROE_XR;\
117 tp_mikroe_cfg.up = TP_MIKROE_YU;\
118 tp_mikroe_cfg.down = TP_MIKROE_YD;
136 uint16_t tp_mikroe_pressure_threshold_lower;
137 uint16_t tp_mikroe_pressure_threshold_upper;
164 #define TP_MIKROE_ADC_CONFIG( cfg ) \
165 cfg->analog_in_cfg_read_x.resolution = ANALOG_IN_RESOLUTION_CMAKE; \
166 cfg->analog_in_cfg_read_y.resolution = ANALOG_IN_RESOLUTION_CMAKE; \
167 cfg->analog_in_cfg_read_x.input_pin = TP_READ_X_CMAKE; \
168 cfg->analog_in_cfg_read_y.input_pin = TP_READ_Y_CMAKE; \
169 cfg->analog_in_cfg_read_x.vref_input = ANALOG_IN_VREF_CMAKE; \
170 cfg->analog_in_cfg_read_y.vref_input = ANALOG_IN_VREF_CMAKE; \
171 cfg->analog_in_cfg_read_x.vref_value = VREF_VALUE; \
172 cfg->analog_in_cfg_read_y.vref_value = VREF_VALUE;
207 gl_set_pen( GL_WHITE, 4 );
213 #define DRAW_ARROW_TOP_LEFT() \
214 gl_clear( GL_BLACK ); \
215 gl_draw_line( 0, 0, 10, 0 ); \
216 gl_draw_line( 0, 0, 0, 10 ); \
217 gl_draw_line( 0, 0, 15, 15 );
223 #define DRAW_ARROW_TOP_RIGHT() \
224 gl_clear( GL_BLACK ); \
225 gl_draw_line( 320, 0, 320, 10 ); \
226 gl_draw_line( 320, 0, 310, 0 ); \
227 gl_draw_line( 320, 0, 305, 15 );
233 #define DRAW_ARROW_BOTTOM_LEFT() \
234 gl_clear( GL_BLACK ); \
235 gl_draw_line( 0, 240, 0, 230 ); \
236 gl_draw_line( 0, 240, 10, 240 ); \
237 gl_draw_line( 0, 240, 15, 225 );
243 #define DRAW_ARROW_BOTTOM_RIGHT() \
244 gl_clear( GL_BLACK ); \
245 gl_draw_line( 320, 240, 320, 230 ); \
246 gl_draw_line( 320, 240, 310, 240 ); \
247 gl_draw_line( 320, 240, 305, 225 );
452 #endif // _TP_MIKROE_H_
void tp_mikroe_calibrate_point(tp_mikroe_t *ctx, bool calibration_points_uninitialized)
Utility function used for calibration.
Touch Panel Context Object.
Definition: tp.h:222
void tp_mikroe_update_ctx_coords(tp_mikroe_t *ctx, uint16_t x_pos, uint16_t y_pos)
Converts x_pos & y_pos raw ADC values to coordinate values and stores them in 'touch point 0' ctx mem...
void tp_mikroe_set_pressure_threshold_level(tp_mikroe_cfg_t *cfg, tp_mikroe_pressure_threshold_t pressure)
Utility function used for setting threshold levels.
Analog input driver configuration structure.
Definition: drv_analog_in.h:106
API for Analog input driver.
tp_event_t tp_mikroe_press_detect(tp_mikroe_t *ctx)
Press detection function.
Analog input driver context structure, consisted of the following fields :
Definition: drv_analog_in.h:126
API for Digital input driver.
tp_event_t
Touch Panel Event Code Definition.
Definition: tp.h:79
tp_err_t tp_mikroe_init_tp(tp_mikroe_t *ctx, tp_mikroe_cfg_t *cfg, tp_drv_t *drv)
TP_MIKROE Touch Panel initialization. This function initializes TP_MIKROE context object using config...
void tp_mikroe_press_coordinates(tp_mikroe_t *ctx, tp_touch_coord_t *touch_item)
Copies touch information from context object to touch item object.
void tp_mikroe_gesture(tp_mikroe_t *ctx, tp_event_t *event)
Read gesture information.
void tp_mikroe_set_threshold(tp_mikroe_t *ctx, uint16_t threshold_upper, uint16_t threshold_lower)
Utility function used for setting threshold levels.
char tp_mikroe_pressure_level_detect(tp_mikroe_t *ctx)
Function used for checking pressure levels.
tp_err_t
Touch Panel Error Code Definition.
Definition: tp.h:63
Touch Point Object Definition.
Definition: tp.h:135
tp_rotate_t
Touch Panel Placement (Orientation) Definition.
Definition: tp.h:115
Pressure threshold data structure.
Definition: tp_mikroe.h:134
tp_err_t tp_mikroe_process(tp_mikroe_t *ctx)
Function processing events.
void tp_mikroe_default_cfg_adc(tp_mikroe_t *ctx)
TP_MIKROE ADC pin configuration function.
hal_pin_name_t pin_name_t
Definition: drv_name.h:73
TP_MIKROE Context Object.
Definition: tp_mikroe.h:177
API for Digital output driver.
void tp_mikroe_calibrate(tp_t *tp_instance)
Calibrates variables used for converting raw TP_MIKROE ADC values to pixel coordinates.
Calibration data structure.
Definition: tp_mikroe.h:123
void tp_mikroe_set_calibration_data(tp_mikroe_t *ctx, const tp_mikroe_calibration_data_t *cdata)
Utility function used for setting calibration data.
TP_MIKROE Configuration Object.
Definition: tp_mikroe.h:143
void tp_mikroe_cfg_setup(tp_mikroe_cfg_t *cfg)
TP_MIKROE Configuration setup Function.
Touch Panel Driver Interface Items.
Definition: tp.h:197
void tp_mikroe_get_calibration_data(tp_mikroe_t *ctx, tp_mikroe_calibration_data_t *cdata)
Utility function used for getting a copy of current calibration data.
bool tp_mikroe_check_pressure(tp_mikroe_t *ctx, uint16_t *x_pos, uint16_t *y_pos)
Function for reading raw ADC data.