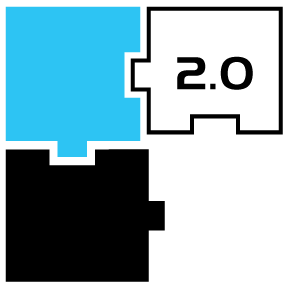 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
58 #define TP_N_TOUCHES_MAX 5
237 uint16_t coord_x_max;
238 uint16_t coord_y_max;
Touch Panel Context Object.
Definition: tp.h:222
tp_touch_id_t
Touch Point ID Definition.
Definition: tp.h:100
tp_event_t tp_press_detect(tp_t *ctx)
Touch Panel Pressure Detector Function.
#define TP_N_TOUCHES_MAX
Touch Panel Touch Limit.
Definition: tp.h:57
void(* tp_gesture_callback_t)(tp_event_t)
Touch Panel Gesture Callback Function.
Definition: tp.h:191
void tp_gesture(tp_t *ctx, tp_event_t *event)
Touch Panel Gesture Check Function.
void(* tp_press_coord_t)(void *, tp_touch_item_t *)
Touch Panel Driver Interface Definition.
Definition: tp.h:166
tp_event_t
Touch Panel Event Code Definition.
Definition: tp.h:79
tp_event_t(* tp_press_det_t)(void *)
Touch Panel Driver Interface Definition.
Definition: tp.h:160
Touch Item Definition.
Definition: tp.h:149
void(* tp_gesture_t)(void *, tp_event_t *)
Touch Panel Driver Interface Definition.
Definition: tp.h:172
void tp_gesture_callback_setup(tp_t *ctx, tp_gesture_callback_t cb)
Touch Panel Callback Setup Function.
tp_err_t(* tp_process_t)(void *)
Touch Panel Driver Interface Definition.
Definition: tp.h:178
tp_err_t
Touch Panel Error Code Definition.
Definition: tp.h:63
Touch Point Object Definition.
Definition: tp.h:135
tp_rotate_t
Touch Panel Placement (Orientation) Definition.
Definition: tp.h:115
void tp_rotate(tp_t *ctx, tp_rotate_t rotate)
Touch Panel Rotate Function.
tp_err_t tp_process(tp_t *ctx)
Touch Panel Process Function.
uint16_t tp_coord_t
Touch Point Coordinates Data Type.
Definition: tp.h:129
void tp_cfg_setup(tp_cfg_t *cfg)
Touch Panel Configuration Function.
tp_err_t tp_init(tp_t *ctx, tp_cfg_t *cfg, tp_drv_t *drv, void *drv_ctx)
Touch Panel Initialization Function.
void(* tp_press_callback_t)(tp_event_t, tp_coord_t, tp_coord_t, tp_touch_id_t)
Touch Panel Touch Callback Function.
Definition: tp.h:184
tp_rotate_t tp_get_orientation(tp_t *ctx)
Touch Panel Orientation Check Function.
void tp_press_callback_setup(tp_t *ctx, tp_press_callback_t cb)
Touch Panel Callback Setup Function.
Touch Panel Size And Placement Configuration Object.
Definition: tp.h:210
void tp_get_size(tp_t *ctx, uint16_t *width, uint16_t *height)
Touch Panel Size Check Function.
Touch Panel Driver Interface Items.
Definition: tp.h:197
tp_err_t tp_press_coordinates(tp_t *ctx, tp_touch_item_t *touch_item)
Touch Panel Pressure Coordinates Check Function.