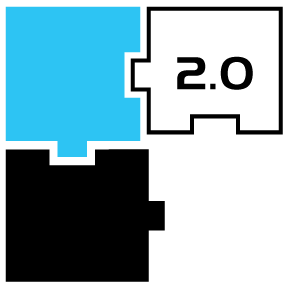 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
50 #include "../../../generic.h"
102 #define ABS(x) (((x)>0)?(x):-(x))
112 #define LOG_MAP_USB_UART(cfg) \
113 cfg.rx_pin = USB_UART_RX; \
114 cfg.tx_pin = USB_UART_TX; \
116 cfg.level = LOG_LEVEL_DEBUG;
121 #define LOG_MAP_MIKROBUS(cfg, mikrobus) \
122 cfg.rx_pin = MIKROBUS(mikrobus, MIKROBUS_RX); \
123 cfg.tx_pin = MIKROBUS(mikrobus, MIKROBUS_TX); \
125 cfg.level = LOG_LEVEL_DEBUG;
225 void log_log (
log_t *
log,
char * prefix,
const code
char * __generic f, ... );
void log_init(log_t *log, log_cfg_t *cfg)
Initializes LOG module.
void log_fatal(log_t *log, const code char *__generic f,...)
FATAL printf function.
void log_debug(log_t *log, const code char *__generic f,...)
DEBUG printf function.
void log_log(log_t *log, char *prefix, const code char *__generic f,...)
Printf function with a variable prefix.
hal_ll_pin_name_t hal_pin_name_t
Definition: hal_target.h:59
void log_clear(log_t *log)
Discards all characters from the output and input buffer.
void log_error(log_t *log, const code char *__generic f,...)
ERROR printf function.
void log_warning(log_t *log, const code char *__generic f,...)
WARNING printf function.
LOG context structure.
Definition: log.h:68
UART driver context structure, consisted of the following fields :
Definition: drv_uart.h:163
double log(double num)
Calculates the natural logarithm - ln( num ).
log_level_t
Log level values.
Definition: log.h:56
int8_t log_read(log_t *log, uint8_t *rx_data_buf, uint8_t max_len)
Reads at most size bytes of data from the device into buffer.
LOG init configuration structure.
Definition: log.h:77
void log_printf(log_t *log, const code char *__generic f,...)
Printf function.
void log_info(log_t *log, const code char *__generic f,...)
INFO printf function.