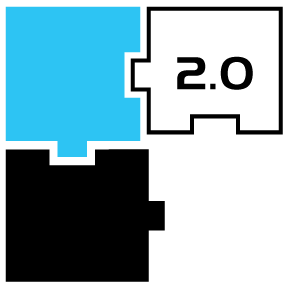 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
63 handle_t *hal_uart_handle;
64 handle_t *drv_uart_handle;
190 uint8_t *tx_ring_buffer;
191 uint8_t *rx_ring_buffer;
193 bool is_tx_irq_enabled;
194 bool is_rx_irq_enabled;
414 size_t hal_uart_write( handle_t *handle, uint8_t *buffer,
size_t size );
477 size_t hal_uart_read( handle_t *handle, uint8_t *buffer,
size_t size );
536 #endif // _HAL_UART_H_
size_t hal_uart_read(handle_t *handle, uint8_t *buffer, size_t size)
Read data from UART.
Definition: hal_uart.h:105
size_t hal_uart_print(handle_t *handle, char *text)
Print the string to UART.
Definition: hal_uart.h:97
Definition: hal_uart.h:76
Definition: hal_uart.h:72
Definition: hal_uart.h:84
Definition: hal_uart.h:122
Definition: hal_uart.h:118
Definition: hal_uart.h:94
hal_uart_err_t
Definition: hal_uart.h:70
UART HAL context structure, consisted of the following fields :
Definition: hal_uart.h:183
size_t hal_uart_bytes_available(hal_uart_t *hal_obj)
Check number of data available to read.
Definition: hal_uart.h:117
err_t hal_uart_open(handle_t *handle, bool hal_obj_open_state)
Open the UART HAL layer object on selected pins.
Definition: hal_uart.h:93
size_t hal_uart_println(handle_t *handle, char *text)
Print the string to UART and append new line.
HAL target macros and typedefs.
hal_ll_pin_name_t hal_pin_name_t
Definition: hal_target.h:59
Definition: hal_uart.h:106
Definition: hal_uart.h:120
hal_uart_stop_bits_t
Predefined enum values for stop bit selection.
Definition: hal_uart.h:115
Definition: hal_uart.h:119
void hal_uart_clear(hal_uart_t *hal_obj)
Discard all characters from UART buffers.
hal_uart_irq_t
Predefined enum values for interrupt request type.
Definition: hal_uart.h:82
Definition: hal_uart.h:73
hal_uart_parity_t
Predefined enum values for parity selection.
Definition: hal_uart.h:103
err_t hal_uart_set_parity(handle_t *handle, hal_uart_config_t *config)
Set the UART parity.
void hal_uart_set_blocking(handle_t *handle, bool blocking)
Set UART HAL in blocking/non-blocking mode.
size_t hal_uart_write(handle_t *handle, uint8_t *buffer, size_t size)
Write data to UART.
Definition: hal_uart.h:85
err_t hal_uart_set_stop_bits(handle_t *handle, hal_uart_config_t *config)
Set the number of UART stop bits.
hal_uart_data_bits_t
Predefined enum values for data bit selection.
Definition: hal_uart.h:91
Definition: hal_uart.h:74
Definition: hal_uart.h:95
Definition: hal_uart.h:107
Definition: hal_uart.h:109
err_t hal_uart_close(handle_t *handle)
Close UART HAL layer object.
err_t hal_uart_set_baud(handle_t *handle, hal_uart_config_t *config)
Set the UART baud rate.
int32_t err_t
Definition: hal_target.h:63
void hal_uart_configure_default(hal_uart_config_t *config)
Configure UART configuration structure with default values.
Definition: hal_uart.h:60
err_t hal_uart_set_data_bits(handle_t *handle, hal_uart_config_t *config)
Set the number of UART data bits.
UART HAL init configuration structure, consisted of the following fields :
Definition: hal_uart.h:157