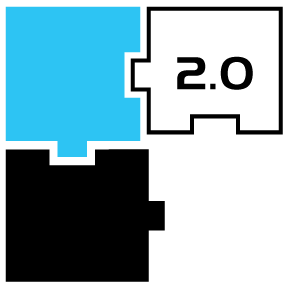 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
44 #ifndef _HAL_ONE_WIRE_H_
45 #define _HAL_ONE_WIRE_H_
652 #endif // _HAL_ONE_WIRE_H_
err_t hal_one_wire_skip_rom(hal_one_wire_t *obj)
Access device's level functions without transmitting ROM information.
Definition: hal_one_wire.h:77
err_t hal_one_wire_write_byte(hal_one_wire_t *obj, uint8_t *write_data_buffer, size_t write_data_length)
Writes byte to One Wire bus.
void one_wire_timing_value_j(void)
Configures device-specific timing "j" sequence value.
err_t hal_one_wire_match_rom(hal_one_wire_t *obj, hal_one_wire_rom_address_t *device_rom_address)
Select a specific One Wire capable device on bus.
void one_wire_timing_value_b(void)
Configures device-specific timing "b" sequence value.
Definition: hal_one_wire.h:76
void one_wire_timing_value_h(void)
Configures device-specific timing "h" sequence value.
void one_wire_timing_value_i(void)
Configures device-specific timing "i" sequence value.
err_t hal_one_wire_search_next_device(hal_one_wire_t *obj, hal_one_wire_rom_address_t *one_wire_device_list)
Search One Wire capable devices on bus.
HAL target macros and typedefs.
hal_ll_pin_name_t hal_pin_name_t
Definition: hal_target.h:59
void one_wire_timing_value_c(void)
Configures device-specific timing "c" sequence value.
err_t hal_one_wire_reset(hal_one_wire_t *obj)
Resets One Wire bus.
err_t hal_one_wire_read_byte(hal_one_wire_t *obj, uint8_t *read_data_buffer, size_t read_data_length)
Reads byte from One Wire bus.
err_t hal_one_wire_search_first_device(hal_one_wire_t *obj, hal_one_wire_rom_address_t *one_wire_device_list)
Search One Wire capable device on bus.
Structure for storing One Wire device address.
Definition: hal_one_wire.h:56
err_t hal_one_wire_read_rom(hal_one_wire_t *obj, hal_one_wire_rom_address_t *device_rom_address)
Reads device's ROM information.
void one_wire_timing_value_e(void)
Configures device-specific timing "e" sequence value.
void hal_one_wire_configure_default(hal_one_wire_t *obj)
Configures One Wire HAL configuration structure.
void one_wire_timing_value_a(void)
Configures device-specific timing "a" sequence value.
One Wire HAL initialization configuration structure.
Definition: hal_one_wire.h:67
void one_wire_timing_value_f(void)
Configures device-specific timing "f" sequence value.
int32_t err_t
Definition: hal_target.h:63
err_t hal_one_wire_open(hal_one_wire_t *obj)
Opens One Wire HAL object.
hal_one_wire_err_t
Definition: hal_one_wire.h:75
void one_wire_timing_value_d(void)
Configures device-specific timing "d" sequence value.