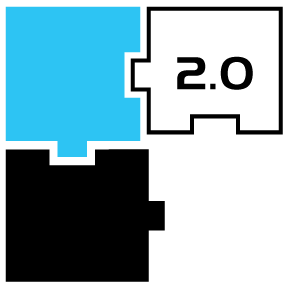 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
63 handle_t *hal_adc_handle;
64 handle_t *drv_adc_handle;
362 #endif // _HAL_ADC_H_
err_t hal_adc_open(handle_t *handle, bool hal_obj_open_state)
Open the ADC HAL layer object on selected pin.
err_t hal_adc_read_voltage(handle_t *handle, float *readDatabuf)
Read analog voltage value on pin.
void hal_adc_set_vref_value(handle_t *handle, hal_adc_config_t *config)
Set ADC HAL reference voltage value to desired value.
hal_adc_vref_t
Definition: hal_adc.h:101
HAL target macros and typedefs.
err_t hal_adc_read(handle_t *handle, uint16_t *readDatabuf)
Read analog value on pin.
ADC HAL initialization configuration structure, consisted of the following fields :
Definition: hal_adc.h:131
Definition: hal_adc.h:104
hal_ll_pin_name_t hal_pin_name_t
Definition: hal_target.h:59
Definition: hal_adc.h:103
Definition: hal_adc.h:105
void hal_adc_configure_default(hal_adc_config_t *config)
Configure ADC HAL configuration structure..
ADC HAL level handle.
Definition: hal_adc.h:60
hal_adc_resolution_t
Definition: hal_adc.h:85
err_t hal_adc_close(handle_t *handle)
Close ADC HAL layer object.
err_t hal_adc_set_vref_input(handle_t *handle, hal_adc_config_t *config)
Set ADC HAL reference voltage source to desired value.
err_t hal_adc_set_resolution(handle_t *handle, hal_adc_config_t *config)
Set ADC HAL sample resolution.
ADC HAL context structure, consisted of the following fields :
Definition: hal_adc.h:149
int32_t err_t
Definition: hal_target.h:63
hal_adc_err_t
Definition: hal_adc.h:70