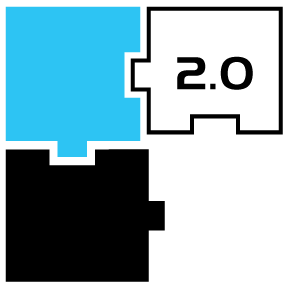 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
148 uint16_t display_width;
149 uint16_t display_height;
161 #endif // _GL_TYPES_H_
Definition: gl_types.h:87
Definition: gl_types.h:61
gl_brush_style_t
Definition: gl_types.h:57
Definition: gl_types.h:74
The context structure for storing driver configuration.
Definition: gl_types.h:145
int32_t gl_long_int_t
Definition: gl_types.h:92
Definition: gl_types.h:76
Definition: gl_types.h:85
The context structure for storing rectangle by its top left point and width and height (in pixels).
Definition: gl_types.h:128
Definition: gl_types.h:73
void(* gl_end_frame_t)()
Definition: gl_types.h:139
gl_image_format_t
Definition: gl_types.h:71
Definition: gl_types.h:75
int16_t gl_coord_t
Definition: gl_types.h:102
Definition: gl_types.h:62
The context structure for storing coordinates of the point.
Definition: gl_types.h:108
void(* gl_fill_t)(gl_rectangle_t *rect, gl_color_t color)
Definition: gl_types.h:136
uint32_t gl_long_uint_t
Definition: gl_types.h:93
Definition: gl_types.h:77
uint16_t gl_color_t
Definition: gl_colors.h:55
Definition: gl_types.h:59
int16_t gl_int_t
Definition: gl_types.h:90
Definition: gl_types.h:86
Definition: gl_types.h:60
gl_font_orientation_t
Definition: gl_types.h:83
uint16_t gl_uint_t
Definition: gl_types.h:91
uint16_t gl_angle_t
Definition: gl_types.h:95
void(* gl_begin_frame_t)(gl_rectangle_t *rect)
Definition: gl_types.h:137
Definition of colors. Here predefined colors can be found.
void(* gl_frame_data_t)(gl_color_t color)
Definition: gl_types.h:138
The context structure for storing width and height in number of pixels on the screen.
Definition: gl_types.h:118