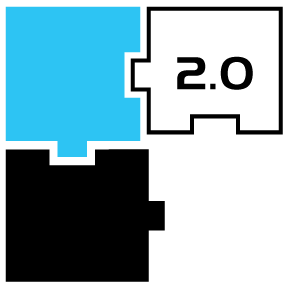 |
mikroSDK Reference Manual
|
void gl_set_pen_color(gl_color_t color)
Sets the active pen's color.
gl_brush_style_t
Definition: gl_types.h:57
void gl_set_font_background_color(gl_color_t background)
Sets the active background color for texts.
void gl_set_font_orientation(gl_font_orientation_t orientation)
Sets the active font orientation.
void gl_set_font(const uint8_t *font)
Initialize the active font.
The context structure for storing driver configuration.
Definition: gl_types.h:145
void gl_set_brush_color(gl_color_t color)
Sets active brush color.
void gl_set_brush_color_from(gl_color_t color)
Sets the active start color.
void gl_set_pen_width(uint16_t width)
Sets the active pen width.
uint16_t gl_get_inner_pen()
Returns the inner width of active pen.
uint16_t gl_get_screen_height()
Returns the height of the display.
Declaration of types for Graphic Library.
void gl_set_driver(gl_driver_t *driver)
Sets the driver to the active state and enables drawing on whole display.
uint16_t gl_get_screen_width()
Returns the width of the display.
bool gl_set_crop_borders(gl_coord_t left, gl_coord_t right, gl_coord_t top, gl_coord_t bottom)
Initialize borders for drawing on display.
uint16_t gl_get_outer_pen()
Returns the outer width of active pen.
API for different shape drawing.
void gl_clear_inside_borders(gl_color_t color)
Paint display within crop borders.
int16_t gl_coord_t
Definition: gl_types.h:102
void gl_set_font_background(bool enable)
Sets active indicator for text background.
void gl_set_brush_style(gl_brush_style_t style)
Sets active brush style.
void gl_clear(gl_color_t color)
Paint whole display.
void gl_set_brush_color_to(gl_color_t color)
Sets the active end color.
uint16_t gl_color_t
Definition: gl_colors.h:55
gl_font_orientation_t
Definition: gl_types.h:83
void gl_set_pen(gl_color_t color, uint16_t width)
Sets the active pen width and color.
void gl_set_outer_pen(uint16_t width_outside_object)
Sets width of outer part of active pen.
Definition of colors. Here predefined colors can be found.
void gl_set_inner_pen(uint16_t width_inside_object)
Sets width of inner part of active pen.