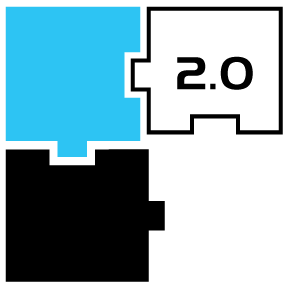 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
44 #ifndef _FILE_SYSTEM_H_
45 #define _FILE_SYSTEM_H_
51 #include "physical_drive.h"
55 #define NOP() asm ("nop")
78 #define FS_MAX_VOLUMES ((uint8_t)5)
85 #define FS_MAX_OBJECTS ((uint8_t)10)
99 #define FSS_OK ((uint8_t)0)
104 #define FSS_GENERAL_ERROR ((uint8_t)1)
109 #define FSS_DRIVE_FULL ((uint8_t)2)
114 #define FSS_END_OF_FILE ((uint8_t)3)
119 #define FSS_END_OF_DIRECTORY ((uint8_t)4)
124 #define FSS_NOT_IMPLEMENTED ((uint8_t)5)
143 #define FS_FILE_READ 0x01
148 #define FS_FILE_WRITE 0x02
153 #define FS_FILE_OPEN_EXISTING 0x00
158 #define FS_FILE_CREATE_NEW 0x04
163 #define FS_FILE_CREATE_ALWAYS 0x08
168 #define FS_FILE_OPEN_ALWAYS 0x10
173 #define FS_FILE_OPEN_APPEND 0x30
253 uint8_t object_count;
434 #endif // !_FILE_SYSTEM_H_
fs_file_rw_pointer_t
: file_seek API Starting Offset
Definition: file_system.h:190
Definition: file_system.h:230
fs_status_t file_system_mkdir(const char *__generic_ptr path)
Make new Directory.
Definition: file_system.h:229
Definition: file_system.h:192
Physical Drive Base Data Structure Reference.
Definition: physical_drive.h:127
fs_status_t file_system_format(const char *__generic_ptr path)
Format the Logical Drive.
int8_t fs_status_t
: File System API return value
Definition: file_system.h:183
void * fs_dir_t
: Directory Data specific to a particular File System.
Definition: file_system.h:211
logical_drive_type_t
Logical Drive File System Type Enumerator.
Definition: file_system.h:228
fs_status_t file_system_rename(const char *__generic_ptr old_path, const char *__generic_ptr new_path)
Renames a File or a Directory.
Logical Drive Vector Table.
Definition: fs_common.h:72
Logical Drive Base Data Structure.
Definition: file_system.h:248
Definition: file_system.h:193
fs_status_t file_system_mount(logical_drive_t *const ldrive_base, const char *__generic_ptr path, physical_drive_t *const pdrive_base)
Mount Logical Drive to the File System.
void * fs_file_t
: File data specific to a particular file system.
Definition: file_system.h:202
fs_status_t file_system_remove(const char *__generic_ptr path)
Removes a File or a Directory.
fs_status_t file_system_unmount(const char *__generic_ptr path)
Unmount Logical Drive from the File System.
Definition: file_system.h:191