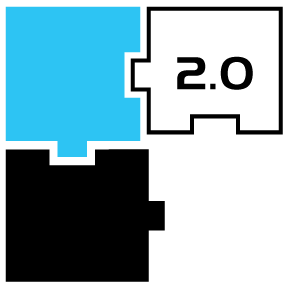 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
44 #ifndef _DRV_I2C_MASTER_H_
45 #define _DRV_I2C_MASTER_H_
104 uint16_t timeout_pass_count;
430 #endif // _DRV_I2C_MASTER_H_
Pin and port name type definitions.
i2c_master_err_t
Definition: drv_i2c_master.h:56
i2c_master_speed_t
Definition: drv_i2c_master.h:65
I2C Master driver context structure, consisted of the following fields :
Definition: drv_i2c_master.h:119
Definition: drv_i2c_master.h:69
err_t i2c_master_write_then_read(i2c_master_t *obj, uint8_t *write_data_buf, size_t len_write_data, uint8_t *read_data_buf, size_t len_read_data)
Write data to I2C bus followed by read.
err_t i2c_master_set_slave_address(i2c_master_t *obj, uint8_t address)
Set I2C Slave address.
err_t i2c_master_write(i2c_master_t *obj, uint8_t *write_data_buf, size_t len_write_data)
Write data to the I2C bus.
err_t i2c_master_read(i2c_master_t *obj, uint8_t *read_data_buf, size_t len_read_data)
Read data from the I2C bus.
Definition: drv_i2c_master.h:59
err_t i2c_master_open(i2c_master_t *obj, i2c_master_config_t *config)
Open the I2C Master driver object.
err_t i2c_master_set_speed(i2c_master_t *obj, uint32_t speed)
Set I2C Master module speed.
API for I2C master HAL layer.
void i2c_master_configure_default(i2c_master_config_t *config)
Configure I2C Master configuration structure.
err_t i2c_master_set_timeout(i2c_master_t *obj, uint16_t timeout_pass_count)
Set I2C Master timeout value.
Definition: drv_i2c_master.h:67
Definition: drv_i2c_master.h:68
Definition: drv_i2c_master.h:58
hal_pin_name_t pin_name_t
Definition: drv_name.h:73
int32_t err_t
Definition: hal_target.h:63
err_t i2c_master_close(i2c_master_t *obj)
Close I2C Master driver object.
I2C Master initialization configuration structure, consisted of the following fields :
Definition: drv_i2c_master.h:95