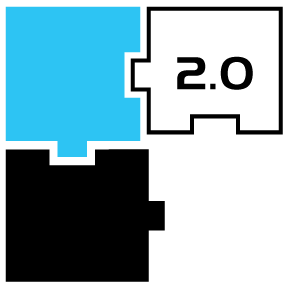 |
mikroSDK Reference Manual
|
Go to the documentation of this file.
44 #ifndef _CONVERSIONS_H_
45 #define _CONVERSIONS_H_
54 #include <me_built_in.h>
324 char *
r_trim(
char *
string );
332 char *
l_trim(
char *
string );
494 #endif // _CONVERSIONS_H_
void uint32_to_str_with_zeros(uint32_t input, char *output)
Converts uint32_t to string with zeros.
void uint32_to_hex(uint32_t input, char *output)
Converts uint32_t to hex value.
void uint8_to_str(uint8_t input, char *output)
Converts uint8_t to string.
void int32_to_str(int32_t input, char *output)
Converts int32_t to string.
uint8_t bcd_to_dec(uint8_t bcdnum)
Converts bcd value to decimal number.
int16_t hex_to_int16(char hex_in[4])
Converts hex into int16.
void uint16_to_str(uint16_t input, char *output)
Converts uint16_t to string.
uint8_t dec_to_bcd(uint8_t decnum)
Converts decimal number to bcd value.
int64_t str_to_int64(char int64_in[20])
Converts string into int64.
uint16_t dec_to_bcd16(uint16_t decnum)
Converts decimal number to bcd value.
float str_to_float(char data_str[20])
Converts string into float.
void int16_to_str_with_zeros(int16_t input, char *output)
Converts int16_t to string with zeros.
void uint32_to_str(uint32_t input, char *output)
Converts uint32_t to string.
void int16_to_hex(int16_t input, char *output)
Converts int16_t to hex value.
int8_t str_to_int8(char int8_in[4])
Converts string into int8.
void uint8_to_str_with_zeros(uint8_t input, char *output)
Converts uint8_t to string with zeros.
int16_t str_to_int16(char int16_in[6])
Converts string into int16.
void int8_to_str_with_zeros(int8_t input, char *output)
Converts int8_t to string with zeros.
uint8_t hex_to_uint8(char hex_in[2])
Converts hex into uint8.
uint8_t float_to_str(float f_num, char *string)
Converts floating point number into string.
void int32_to_str_with_zeros(int32_t input, char *output)
Converts int32_t to string with zeros.
int32_t str_to_int32(char int32_in[11])
Converts string into int32.
int32_t hex_to_int32(char hex_in[8])
Converts hex into int32.
void uint16_to_hex(uint16_t input, char *output)
Converts uint16_t to hex value.
char * l_trim(char *string)
Trims the leading spaces from an array given with *string.
void int8_to_str(int8_t input, char *output)
Converts int8_t to string.
void int32_to_hex(int32_t input, char *output)
Converts int32_t to hex value.
void uint64_to_hex(uint64_t input, char *output)
Converts uint64_t to hex value.
void int64_to_hex(int64_t input, char *output)
Converts int64_t to hex value.
uint32_t hex_to_uint32(char hex_in[8])
Converts hex into uint32.
void uint64_to_str_with_zeros(uint64_t input, char *output)
Converts uint64_t to string with zeros.
int8_t hex_to_int8(char hex_in[2])
Converts hex into int8.
void int64_to_str(int64_t input, char *output)
Converts int64_t to string.
char * r_trim(char *string)
Trims the trailing spaces from an array given with *string.
void uint8_to_hex(uint8_t input, char *output)
Converts uint8_t to hex value.
uint64_t str_to_uint64(char uint64_in[20])
Converts string into uint64.
uint16_t bcd_to_dec16(uint16_t bcdnum)
Converts bcd value to decimal number.
int64_t hex_to_int64(char hex_in[16])
Converts hex into int64.
uint8_t long_double_to_str(long double dnum, char *str)
Converts a long double number into a string.
void int8_to_hex(int8_t input, char *output)
Converts int8_t to hex value.
uint8_t str_to_uint8(char uint8_in[3])
Converts string into uint8.
void int16_to_str(int16_t input, char *output)
Converts int16_t to string.
void uint64_to_str(uint64_t input, char *output)
Converts uint64_t to string.
void int64_to_str_with_zeros(int64_t input, char *output)
Converts int64_t to string with zeros.
uint16_t str_to_uint16(char uint16_in[5])
Converts string into uint16.
void uint16_to_str_with_zeros(uint16_t input, char *output)
Converts uint16_t to string with zeros.
uint16_t hex_to_uint16(char hex_in[4])
Converts hex into uint16.
uint32_t str_to_uint32(char uint32_in[10])
Converts string into uint32.
uint64_t hex_to_uint64(char hex_in[16])
Converts hex into uint64.