SPI T6963C Graphic Lcd Library
The mikroC PRO for PIC32 provides a library for working with Glcds based on TOSHIBA T6963C controller via SPI interface. The Toshiba T6963C is a very popular Lcd controller for the use in small graphics modules. It is capable of controlling displays with a resolution up to 240x128. Because of its low power and small outline it is most suitable for mobile applications such as PDAs, MP3 players or mobile measurement equipment. Although this controller is small, it has a capability of displaying and merging text and graphics and it manages all interfacing signals to the displays Row and Column drivers.

- When using this library with PIC32 family MCUs be aware of their voltage incompatibility with certain number of T6963C based Glcd modules. So, additional external power supply for these modules may be required.
- Glcd size based initialization routines can be found in setup library files located in the Uses folder.
- The user must make sure that used MCU has appropriate ports and pins. If this is not the case the user should adjust initialization routines.
- The library uses the SPI module for communication. The user must initialize the appropriate SPI module before using the SPI T6963C Glcd Library.
- For MCUs with multiple SPI modules it is possible to initialize both of them and then switch by using the
SPI_Set_Active()
routine. See the SPI Library functions. - This Library is designed to work with mikroElektronika's Serial Glcd 240x128 and 240x64 Adapter Boards pinout, see schematic at the bottom of this page for details.
- To use constants located in
__Lib_SPIT6963C_Const.h
file, user must include it the source file :#include "__SPIT6963C.h"
.
Some mikroElektronika's adapter boards have pinout different from T6369C datasheets. Appropriate relations between these labels are given in the table below:
Adapter Board | T6369C datasheet |
---|---|
RS | C/D |
R/W | /RD |
E | /WR |
Library Dependency Tree
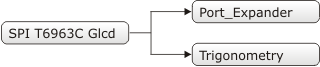
External dependencies of SPI T6963C Graphic Lcd Library
The implementation of SPI T6963C Graphic Lcd Library routines is based on Port Expander Library routines.
External dependencies are the same as Port Expander Library external dependencies.
Library Routines
- SPI_T6963C_config
- SPI_T6963C_writeData
- SPI_T6963C_writeCommand
- SPI_T6963C_setPtr
- SPI_T6963C_Set_Ext_Buffer
- SPI_T6963C_waitReady
- SPI_T6963C_fill
- SPI_T6963C_dot
- SPI_T6963C_Set_Font_Adv
- SPI_T6963C_Set_Font_Ext_Adv
- SPI_T6963C_write_char
- SPI_T6963C_Write_Char_Adv
- SPI_T6963C_write_text
- SPI_T6963C_Write_Text_Adv
- SPI_T6963C_line
- SPI_T6963C_rectangle
- SPI_T6963C_rectangle_round_edges
- SPI_T6963C_rectangle_round_edges_fill
- SPI_T6963C_box
- SPI_T6963C_circle
- SPI_T6963C_circle_fill
- SPI_T6963C_image
- SPI_T6963C_Ext_Image
- SPI_T6963C_PartialImage
- SPI_T6963C_Ext_PartialImage
- SPI_T6963C_sprite
- SPI_T6963C_set_cursor
- SPI_T6963C_clearBit
- SPI_T6963C_setBit
- SPI_T6963C_negBit
The following low level library routines are implemented as macros. These macros can be found in the __SPIT6963C.h
header file which is located in the SPI T6963C example projects folders.
- SPI_T6963C_displayGrPanel
- SPI_T6963C_displayTxtPanel
- SPI_T6963C_setGrPanel
- SPI_T6963C_setTxtPanel
- SPI_T6963C_panelFill
- SPI_T6963C_grFill
- SPI_T6963C_txtFill
- SPI_T6963C_cursor_height
- SPI_T6963C_graphics
- SPI_T6963C_text
- SPI_T6963C_cursor
- SPI_T6963C_cursor_blink
SPI_T6963C_config
Prototype |
void SPI_T6963C_config(unsigned int width, unsigned char height, unsigned char fntW, char DeviceAddress, unsigned char wr, unsigned char rd, unsigned char cd, unsigned char rst); |
---|---|
Description |
Initializes T6963C Graphic Lcd controller. Display RAM organization: +---------------------+ /\ + GRAPHICS PANEL #0 + | + + | + + | + + | +---------------------+ | PANEL 0 + TEXT PANEL #0 + | + + \/ +---------------------+ /\ + GRAPHICS PANEL #1 + | + + | + + | + + | +---------------------+ | PANEL 1 + TEXT PANEL #1 + | + + | +---------------------+ \/ |
Parameters |
|
Returns |
Nothing. |
Requires |
Global variables :
|
Example |
// Port Expander module connections sbit SPExpanderRST at LATF0_bit; sbit SPExpanderCS at LATF1_bit; sbit SPExpanderRST_Direction at TRISF0_bit; sbit SPExpanderCS_Direction at TRISF1_bit; // End Port Expander module connections ... // Initialize SPI module SPI1_Init(); SPI_T6963C_Config(240, 64, 8, 0, 0, 1, 3, 4); |
Notes |
None. |
SPI_T6963C_writeData
Prototype |
void SPI_T6963C_writeData(unsigned char data_); |
---|---|
Description |
Writes data to T6963C controller via SPI interface. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_writeData(data_); |
Notes |
None. |
SPI_T6963C_writeCommand
Prototype |
void SPI_T6963C_writeCommand(unsigned char data_); |
---|---|
Description |
Writes command to T6963C controller via SPI interface. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_writeCommand(SPI_T6963C_CURSOR_POINTER_SET); |
Notes |
None. |
SPI_T6963C_setPtr
Prototype |
void SPI_T6963C_setPtr(unsigned int p, unsigned char c); |
---|---|
Description |
Sets the memory pointer |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_setPtr(SPI_T6963C_grHomeAddr + start, SPI_T6963C_ADDRESS_POINTER_SET); |
Notes |
None. |
SPI_T6963C_Set_Ext_Buffer
Prototype |
void SPI_T6963C_Set_Ext_Buffer(char* (*getExtDataPtr)(unsigned long offset, unsigned long count, unsigned long *num)); |
---|---|
Returns |
Nothing. |
Description |
Function sets pointer to the user function which manipulates the external resource. Parameters :
|
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
char* ReadExternalBuffer(unsigned long offset, unsigned int count, unsigned int *num){ unsigned long start_sector; unsigned int pos; start_sector = Mmc_Get_File_Write_Sector() + offset/512; pos = (unsigned long)offset%512; if(start_sector == currentSector+1){ Mmc_Multi_Read_Buffer(EXT_BUFFER); currentSector = start_sector; }else if(start_sector != currentSector){ Mmc_Multi_Read_Stop(); Mmc_Multi_Read_Start(start_sector); Mmc_Multi_Read_Buffer(EXT_BUFFER); currentSector = start_sector; } if(count>512-pos){ *num = 512-pos; } else *num = count; return EXT_BUFFER+pos; } SPI_T6963C_Set_Ext_Buffer(ReadExternalBuffer); |
SPI_T6963C_waitReady
Prototype |
void SPI_T6963C_waitReady(); |
---|---|
Description |
Pools the status byte, and loops until Toshiba Glcd module is ready. |
Parameters |
None. |
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_waitReady(); |
Notes |
None. |
SPI_T6963C_fill
Prototype |
void SPI_T6963C_fill(unsigned char v, unsigned int start, unsigned int len); |
---|---|
Description |
Fills controller memory block with given byte. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_fill(0x33,0x00FF,0x000F); |
Notes |
None. |
SPI_T6963C_dot
Prototype |
void SPI_T6963C_dot(int x, int y, unsigned char color); |
---|---|
Description |
Draws a dot in the current graphic panel of Glcd at coordinates (x, y). |
Parameters |
|
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_dot(x0, y0, SPI_T6963C_BLACK); |
Notes |
None. |
SPI_T6963C_Set_Font_Adv
Prototype |
void SPI_T6963C_Set_Font_Adv(const char *activeFont, unsigned char font_color, char font_orientation); |
---|---|
Description |
Sets font that will be used with SPI_T6963C_Write_Char_Adv and SPI_T6963C_Write_Text_Adv routines. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_Set_Font_Adv(&myfont, 1, 0); |
Notes |
None. |
SPI_T6963C_Set_Ext_Font_Adv
Prototype |
void SPI_T6963C_Set_Ext_Font_Adv(unsigned long activeFont, unsigned int font_color, char font_orientation); |
---|---|
Description |
Sets font that will be used with SPI_T6963C_Write_Char_Adv and SPI_T6963C_Write_Text_Adv routines. Font is located in an external resource. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_Set_Ext_Font_Adv(173296, 0, 0); |
Notes |
None. |
SPI_T6963C_write_char
Prototype |
void SPI_T6963C_write_char(unsigned char c, unsigned char x, unsigned char y, unsigned char mode); |
---|---|
Description |
Writes a char in the current text panel of Glcd at coordinates (x, y). |
Parameters |
Mode parameter explanation:
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_write_char("A",22,23,SPI_T6963C_ROM_MODE_AND); |
Notes |
None. |
SPI_T6963C_write_char_adv
Prototype |
void SPI_T6963C_write_char_adv(unsigned char c, unsigned int x, unsigned int y); |
---|---|
Description |
Writes a char in the current text panel of Glcd at coordinates (x, y). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_write_char_adv('A',22,23); |
Notes |
None. |
SPI_T6963C_write_text
Prototype |
void SPI_T6963C_write_text(unsigned char *str, unsigned char x, unsigned char y, unsigned char mode); |
---|---|
Description |
Writes text in the current text panel of Glcd at coordinates (x, y). |
Parameters |
Mode parameter explanation:
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_write_text("Glcd LIBRARY DEMO, WELCOME !", 0, 0, SPI_T6963C_ROM_MODE_XOR); |
Notes |
None. |
SPI_T6963C_Write_Text_Adv
Prototype |
void SPI_T6963C_Write_Text_Adv(unsigned char *text, unsigned int x, unsigned int y); |
---|---|
Description |
Writes text in the current text panel of Glcd at coordinates (x, y). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_Write_Text_Adv("Glcd LIBRARY DEMO, WELCOME !", 0, 0); |
Notes |
None. |
SPI_T6963C_line
Prototype |
void SPI_T6963C_line(int x0, int y0, int x1, int y1, unsigned char pcolor); |
---|---|
Description |
Draws a line from (x0, y0) to (x1, y1). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_line(0, 0, 239, 127, SPI_T6963C_WHITE); |
Notes |
None. |
SPI_T6963C_rectangle
Prototype |
void SPI_T6963C_rectangle(int x0, int y0, int x1, int y1, unsigned char pcolor); |
---|---|
Description |
Draws a rectangle on Glcd. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_rectangle(20, 20, 219, 107, SPI_T6963C_WHITE); |
Notes |
None. |
SPI_T6963C_rectangle_round_edges
Prototype |
void SPI_T6963C_rectangle_round_edges(int x0, int y0, int x1, int y1, int round_radius, unsigned char pcolor); |
---|---|
Description |
Draws a rounded edge rectangle on Glcd. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_rectangle_round_edges(20, 20, 219, 107, 12, SPI_T6963C_WHITE); |
Notes |
None. |
SPI_T6963C_rectangle_round_edges_fill
Prototype |
void SPI_T6963C_rectangle_round_edges_fill(int x0, int y0, int x1, int y1, int round_radius, unsigned char pcolor); |
---|---|
Description |
Draws a filled rounded edge rectangle on Glcd. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_rectangle_round_edges_fill(20, 20, 219, 107, 12, SPI_T6963C_WHITE); |
Notes |
None. |
SPI_T6963C_box
Prototype |
void SPI_T6963C_box(int x0, int y0, int x1, int y1, unsigned char pcolor); |
---|---|
Description |
Draws a box on the Glcd |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_box(0, 119, 239, 127, SPI_T6963C_WHITE); |
Notes |
None. |
SPI_T6963C_circle
Prototype |
void SPI_T6963C_circle(int x, int y, long r, unsigned char pcolor); |
---|---|
Description |
Draws a circle on the Glcd. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_circle(120, 64, 110, SPI_T6963C_WHITE); |
Notes |
None. |
SPI_T6963C_circle_fill
Prototype |
void SPI_T6963C_circle_fill(int x, int y, long r, unsigned char pcolor); |
---|---|
Description |
Draws a filled circle on the Glcd. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_circle_fill(120, 64, 110, SPI_T6963C_WHITE); |
Notes |
None. |
SPI_T6963C_image
Prototype |
void SPI_T6963C_image(const code char *pic); |
---|---|
Description |
Displays bitmap on Glcd. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_image(my_image); |
Notes |
Image dimension must match the display dimension. Use the integrated Glcd Bitmap Editor (menu option Tools › Glcd Bitmap Editor) to convert image to a constant array suitable for displaying on Glcd. |
SPI_T6963C_Ext_Image
Prototype |
void SPI_T6963C_Ext_Image(unsigned long image); |
---|---|
Description |
Displays a bitmap from an external resource. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_Ext_image(153608); |
Notes |
Image dimension must match the display dimension. Use the integrated Glcd Bitmap Editor (menu option Tools › Glcd Bitmap Editor) to convert image to a constant array suitable for displaying on Glcd. |
SPI_T6963C_PartialImage
Prototype |
void SPI_T6963C_PartialImage(unsigned int x_left, unsigned int y_top, unsigned int width, unsigned int height, unsigned int picture_width, unsigned int picture_height, code const unsigned short * image); |
---|---|
Description |
Displays a partial area of the image on a desired location. |
Parameters |
|
Description |
Displays a partial area of the image on a desired location. |
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// Draws a 10x15 part of the image starting from the upper left corner on the coordinate (10,12). Original image size is 16x32. SPI_T6963C_PartialImage(10, 12, 10, 15, 16, 32, image); |
Notes |
Image dimension must match the display dimension. Use the integrated Glcd Bitmap Editor (menu option Tools › Glcd Bitmap Editor) to convert image to a constant array suitable for displaying on Glcd. |
SPI_T6963C_Ext_PartialImage
Prototype |
void SPI_T6963C_Ext_PartialImage(unsigned int x_left, unsigned int y_top, unsigned int width, unsigned int height, unsigned int picture_width, unsigned int picture_height, unsigned long image); |
---|---|
Description |
Displays a partial area of the image (from an external resource) on a desired location. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_Ext_PartialImage(10, 12, 10, 15, 16, 32, 0); |
Notes |
Image dimension must match the display dimension. Use the integrated Glcd Bitmap Editor (menu option Tools › Glcd Bitmap Editor) to convert image to a constant array suitable for displaying on Glcd. |
SPI_T6963C_sprite
Prototype |
void SPI_T6963C_sprite(unsigned char px, unsigned char py, const code char *pic, unsigned char sx, unsigned char sy); |
---|---|
Description |
Fills graphic rectangle area (px, py) to (px+sx, py+sy) with custom size picture. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_sprite(76, 4, einstein, 88, 119); // draw a sprite |
Notes |
If |
SPI_T6963C_set_cursor
Prototype |
void SPI_T6963C_set_cursor(unsigned char x, unsigned char y); |
---|---|
Description |
Sets cursor to row x and column y. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_set_cursor(cposx, cposy); |
Notes |
None. |
SPI_T6963C_clearBit
Prototype |
void SPI_T6963C_clearBit(char b); |
---|---|
Description |
Clears control port bit(s). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// clear bits 0 and 1 on control port SPI_T6963C_clearBit(0x03); |
Notes |
None. |
SPI_T6963C_setBit
Prototype |
void SPI_T6963C_setBit(char b); |
---|---|
Description |
Sets control port bit(s). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// set bits 0 and 1 on control port SPI_T6963C_setBit(0x03); |
Notes |
None. |
SPI_T6963C_negBit
Prototype |
void SPI_T6963C_negBit(char b); |
---|---|
Description |
Negates control port bit(s). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// negate bits 0 and 1 on control port SPI_T6963C_negBit(0x03); |
Notes |
None. |
SPI_T6963C_displayGrPanel
Prototype |
void SPI_T6963C_displayGrPanel(unsigned int n); |
---|---|
Description |
Display selected graphic panel. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// display graphic panel 1 SPI_T6963C_displayGrPanel(1); |
Notes |
None. |
SPI_T6963C_displayTxtPanel
Prototype |
void SPI_T6963C_displayTxtPanel(unsigned int n); |
---|---|
Description |
Display selected text panel. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// display text panel 1 SPI_T6963C_displayTxtPanel(1); |
Notes |
None. |
SPI_T6963C_setGrPanel
Prototype |
void SPI_T6963C_setGrPanel(unsigned int n); |
---|---|
Description |
Compute start address for selected graphic panel and set appropriate internal pointers. All subsequent graphic operations will be preformed at this graphic panel. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// set graphic panel 1 as current graphic panel. SPI_T6963C_setGrPanel(1); |
Notes |
None. |
SPI_T6963C_setTxtPanel
Prototype |
void SPI_T6963C_setTxtPanel(unsigned int n); |
---|---|
Description |
Compute start address for selected text panel and set appropriate internal pointers. All subsequent text operations will be preformed at this text panel. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// set text panel 1 as current text panel. SPI_T6963C_setTxtPanel(1); |
Notes |
None. |
SPI_T6963C_panelFill
Prototype |
void SPI_T6963C_panelFill(unsigned char v); |
---|---|
Description |
Fill current panel in full (graphic+text) with appropriate value (0 to clear). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
clear current panel SPI_T6963C_panelFill(0); |
Notes |
None. |
SPI_T6963C_grFill
Prototype |
void SPI_T6963C_grFill(unsigned char v); |
---|---|
Description |
Fill current graphic panel with appropriate value (0 to clear). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// clear current graphic panel SPI_T6963C_grFill(0); |
Notes |
None. |
SPI_T6963C_txtFill
Prototype |
void SPI_T6963C_txtFill(unsigned char v); |
---|---|
Description |
Fill current text panel with appropriate value (0 to clear). |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// clear current text panel SPI_T6963C_txtFill(0); |
Notes |
None. |
SPI_T6963C_cursor_height
Prototype |
void SPI_T6963C_cursor_height(unsigned char n); |
---|---|
Description |
Set cursor size. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
SPI_T6963C_cursor_height(7); |
Notes |
None. |
SPI_T6963C_graphics
Prototype |
void SPI_T6963C_graphics(unsigned int n); |
---|---|
Description |
Enable/disable graphic displaying. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// enable graphic displaying SPI_T6963C_graphics(1); |
Notes |
None. |
SPI_T6963C_text
Prototype |
void SPI_T6963C_text(unsigned int n); |
---|---|
Description |
Enable/disable text displaying. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// enable text displaying SPI_T6963C_text(1); |
Notes |
None. |
SPI_T6963C_cursor
Prototype |
void SPI_T6963C_cursor(unsigned int n); |
---|---|
Description |
Set cursor on/off. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// set cursor on SPI_T6963C_cursor(1); |
Notes |
None. |
SPI_T6963C_cursor_blink
Prototype |
void SPI_T6963C_cursor_blink(unsigned int n); |
---|---|
Description |
Enable/disable cursor blinking. |
Parameters |
|
Returns |
Nothing. |
Requires |
Toshiba Glcd module needs to be initialized. See SPI_T6963C_Config routine. |
Example |
// enable cursor blinking SPI_T6963C_cursor_blink(1); |
Notes |
None. |
Library Example
The following drawing demo tests advanced routines of the SPI T6963C Glcd library. Hardware configurations in this example are made for the LV-32MX v6 board and PIC32MX460F512L.
#include "__SPIT6963C.h"
/*
* bitmap pictures stored in ROM
*/
const code char mikroE_240x128_bmp[];
const code char einstein[];
// Port Expander module connections
sbit SPExpanderRST at LATD8_bit;
sbit SPExpanderCS at LATD9_bit;
sbit SPExpanderRST_Direction at TRISD8_bit;
sbit SPExpanderCS_Direction at TRISD9_bit;
// End Port Expander module connections
void main() {
#define COMPLETE_EXAMPLE
#define LINE_DEMO // Uncomment to demonstrate line drawing routines
#define FILL_DEMO // Uncomment to demonstrate fill routines
#define PARTIAL_IMAGE_DEMO // Uncomment to demonstrate partial image routine
char txt1[] = " EINSTEIN WOULD HAVE LIKED mE";
char txt[] = " GLCD LIBRARY DEMO, WELCOME !";
char txt2[] = "Partial image demo!";
unsigned char panel; // Current panel
unsigned int i; // General purpose register
unsigned char curs; // Cursor visibility
unsigned int cposx, cposy; // Cursor x-y position
CHECON = 0x32;
AD1PCFG = 0xFFFF; // initialize AN pins as digital
TRISB8_bit = 1; // Set RB8 as input
TRISB9_bit = 1; // Set RB9 as input
TRISB10_bit = 1; // Set RB10 as input
TRISB11_bit = 1; // Set RB11 as input
TRISB12_bit = 1; // Set RB12 as input
TRISB13_bit = 1; // Set RB13 as input
// If Port Expander Library uses SPI1 module
// Initialize SPI module used with PortExpander
SPI2_Init_Advanced(_SPI_MASTER,_SPI_8_BIT, 4, _SPI_SS_DISABLE,_SPI_DATA_SAMPLE_MIDDLE,_SPI_CLK_IDLE_LOW,_SPI_ACTIVE_2_IDLE);
/*
* init display for 240 pixel width and 128 pixel height
* 8 bits character width
* data bus on MCP23S17 portB
* control bus on MCP23S17 portA
* bit 2 is !WR
* bit 1 is !RD
* bit 0 is !CD
* bit 4 is RST
* chip enable, reverse on, 8x8 font internaly set in library
*/
SPI_T6963C_Config(240, 128, 8, 0, 2, 1, 0, 4);
Delay_ms(1000);
/*
* Enable both graphics and text display at the same time
*/
SPI_T6963C_graphics(1);
SPI_T6963C_text(1);
panel = 0;
i = 0;
curs = 0;
cposx = cposy = 0;
/*
* Text messages
*/
SPI_T6963C_write_text(txt, 0, 0, SPI_T6963C_ROM_MODE_XOR);
SPI_T6963C_write_text(txt1, 0, 15, SPI_T6963C_ROM_MODE_XOR);
/*
* Cursor
*/
SPI_T6963C_cursor_height(8); // 8 pixel height
SPI_T6963C_set_cursor(0, 0); // move cursor to top left
SPI_T6963C_cursor(0); // cursor off
/*
* Draw solid boxes
*/
SPI_T6963C_box(0, 0, 239, 8, SPI_T6963C_WHITE);
SPI_T6963C_box(0, 119, 239, 127, SPI_T6963C_WHITE);
/*
* Draw rectangles
*/
#ifdef LINE_DEMO
SPI_T6963C_rectangle(0, 0, 239, 127, SPI_T6963C_WHITE);
SPI_T6963C_rectangle(20, 20, 219, 107, SPI_T6963C_WHITE);
SPI_T6963C_rectangle(40, 40, 199, 87, SPI_T6963C_WHITE);
SPI_T6963C_rectangle(60, 60, 179, 67, SPI_T6963C_WHITE);
#endif
/*
* Draw rounded edge rectangle
*/
#ifdef LINE_DEMO
SPI_T6963C_Rectangle_Round_Edges(10, 10, 229, 117, 12, SPI_T6963C_WHITE);
SPI_T6963C_Rectangle_Round_Edges(30, 30, 209, 97, 12, SPI_T6963C_WHITE);
SPI_T6963C_Rectangle_Round_Edges(50, 50, 189, 77, 12, SPI_T6963C_WHITE);
#endif
/*
* Draw filled rounded edge rectangle
*/
#ifdef FILL_DEMO
SPI_T6963C_Rectangle_Round_Edges_Fill(10, 10, 229, 117, 12, SPI_T6963C_WHITE);
SPI_T6963C_Rectangle_Round_Edges_Fill(20, 20, 219, 107, 12, SPI_T6963C_BLACK);
SPI_T6963C_Rectangle_Round_Edges_Fill(30, 30, 209, 97, 12, SPI_T6963C_WHITE);
SPI_T6963C_Rectangle_Round_Edges_Fill(40, 40, 199, 87, 12, SPI_T6963C_BLACK);
SPI_T6963C_Rectangle_Round_Edges_Fill(50, 50, 189, 77, 12, SPI_T6963C_WHITE);
#endif
/*
* Draw a cross
*/
#ifdef LINE_DEMO
SPI_T6963C_line(0, 0, 239, 127, SPI_T6963C_WHITE);
SPI_T6963C_line(0, 127, 239, 0, SPI_T6963C_WHITE);
#endif
/*
* Draw circles
*/
#ifdef LINE_DEMO
SPI_T6963C_circle(120, 64, 10, SPI_T6963C_WHITE);
SPI_T6963C_circle(120, 64, 30, SPI_T6963C_WHITE);
SPI_T6963C_circle(120, 64, 50, SPI_T6963C_WHITE);
SPI_T6963C_circle(120, 64, 70, SPI_T6963C_WHITE);
SPI_T6963C_circle(120, 64, 90, SPI_T6963C_WHITE);
SPI_T6963C_circle(120, 64, 110, SPI_T6963C_WHITE);
SPI_T6963C_circle(120, 64, 130, SPI_T6963C_WHITE);
#endif
/*
* Draw filled circles
*/
#ifdef FILL_DEMO
SPI_T6963C_circle_fill(120, 64, 60, SPI_T6963C_WHITE);
SPI_T6963C_circle_fill(120, 64, 55, SPI_T6963C_BLACK);
SPI_T6963C_circle_fill(120, 64, 50, SPI_T6963C_WHITE);
SPI_T6963C_circle_fill(120, 64, 45, SPI_T6963C_BLACK);
SPI_T6963C_circle_fill(120, 64, 40, SPI_T6963C_WHITE);
SPI_T6963C_circle_fill(120, 64, 35, SPI_T6963C_BLACK);
SPI_T6963C_circle_fill(120, 64, 30, SPI_T6963C_WHITE);
SPI_T6963C_circle_fill(120, 64, 25, SPI_T6963C_BLACK);
SPI_T6963C_circle_fill(120, 64, 20, SPI_T6963C_WHITE);
SPI_T6963C_circle_fill(120, 64, 15, SPI_T6963C_BLACK);
SPI_T6963C_circle_fill(120, 64, 10, SPI_T6963C_WHITE);
SPI_T6963C_circle_fill(120, 64, 5, SPI_T6963C_BLACK);
#endif
Delay_ms(1000);
SPI_T6963C_sprite(76, 4, einstein, 88, 119); // Draw a sprite
Delay_ms(1000);
SPI_T6963C_setGrPanel(1); // Select other graphic panel
SPI_T6963C_image(mikroE_240x128_bmp);
SPI_T6963C_displayGrPanel(1);
Delay_ms(1000);
#ifdef PARTIAL_IMAGE_DEMO
SPI_T6963C_grFill(0);
SPI_T6963C_PartialImage(0, 0, 64, 64, 240, 128, mikroE_240x128_bmp); // Display partial image
Delay_ms(1000);
SPI_T6963C_graphics(0);
#endif
SPI_T6963C_image(mikroE_240x128_bmp);
SPI_T6963C_graphics(1);
SPI_T6963C_displayGrPanel(0);
for(;;) { // Endless loop
/*
* If RB8 is pressed, display only graphic panel
*/
if(RB8_bit) {
SPI_T6963C_graphics(1);
SPI_T6963C_text(0);
Delay_ms(300);
}
#ifdef COMPLETE_EXAMPLE
/*
* If RB9 is pressed, toggle the display between graphic panel 0 and graphic panel 1
*/
else if(RB9_bit) {
panel++;
panel &= 1;
SPI_T6963C_displayGrPanel(panel);
Delay_ms(300);
}
#endif
/*
* If RB10 is pressed, display only text panel
*/
else if(RB10_bit) {
SPI_T6963C_graphics(0);
SPI_T6963C_text(1);
Delay_ms(300);
}
/*
* If RB11 is pressed, display text and graphic panels
*/
else if(RB11_bit) {
SPI_T6963C_graphics(1);
SPI_T6963C_text(1);
Delay_ms(300);
}
/*
* If RB12 is pressed, change cursor
*/
else if(RB12_bit) {
curs++;
if(curs == 3) curs = 0;
switch(curs) {
case 0:
// no cursor
SPI_T6963C_cursor(0);
break;
case 1:
// blinking cursor
SPI_T6963C_cursor(1);
SPI_T6963C_cursor_blink(1);
break;
case 2:
// non blinking cursor
SPI_T6963C_cursor(1);
SPI_T6963C_cursor_blink(0);
break;
}
Delay_ms(300);
}
#ifdef PARTIAL_IMAGE_DEMO
/*
* If RB13 is pressed, perform the "Partial image" demostration
*/
else if(RB13_bit) {
SPI_T6963C_setGrPanel(0);
SPI_T6963C_setTxtPanel(0);
SPI_T6963C_txtFill(0);
SPI_T6963C_setGrPanel(1);
SPI_T6963C_setTxtPanel(0);
SPI_T6963C_graphics(1);
SPI_T6963C_text(1);
SPI_T6963C_displayGrPanel(1);
SPI_T6963C_write_text(txt2, 5, 15, SPI_T6963C_ROM_MODE_XOR);
Delay_1sec();
SPI_T6963C_grFill(0);
SPI_T6963C_PartialImage(0, 0, 64, 64, 240, 128, mikroE_240x128_bmp);
Delay_1sec();
SPI_T6963C_PartialImage(0, 0, 128, 128, 240, 128, mikroE_240x128_bmp);
Delay_1sec();
SPI_T6963C_PartialImage(0, 0, 240, 128, 240, 128, mikroE_240x128_bmp);
Delay_1sec();
SPI_T6963C_txtFill(0);
SPI_T6963C_write_text(txt, 0, 0, SPI_T6963C_ROM_MODE_XOR);
SPI_T6963C_write_text(txt1, 0, 15, SPI_T6963C_ROM_MODE_XOR);
}
#endif
/*
* Move cursor, even if not visible
*/
cposx++;
if(cposx == SPI_T6963C_txtCols) {
cposx = 0;
cposy++;
if(cposy == SPI_T6963C_grHeight / SPI_T6963C_CHARACTER_HEIGHT) {
cposy = 0;
}
}
SPI_T6963C_set_cursor(cposx, cposy);
Delay_ms(100);
}
}
HW Connection
SPI T6963C Glcd HW connection
What do you think about this topic ? Send us feedback!