SPI Lcd Library
The mikroC PRO for PIC32 provides a library for communication with Lcd (with HD44780 compliant controllers) in 4-bit mode via SPI interface.

- When using this library with PIC32 family MCUs be aware of their voltage incompatibility with certain number of Lcd modules.
So, additional external power supply for these modules may be required. - Library uses the SPI module for communication. The user must initialize the appropriate SPI module before using the SPI Lcd Library.
- For MCUs with multiple SPI modules it is possible to initialize all of them and then switch by using the
SPI_Set_Active()
routine. See the SPI Library functions. - This Library is designed to work with the mikroElektronika's Serial Lcd Adapter Board pinout, see schematic at the bottom of this page for details.
Library Dependency Tree
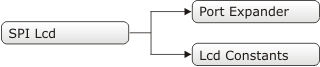
External dependencies of SPI Lcd Library
The implementation of SPI Lcd Library routines is based on Port Expander Library routines.
External dependencies are the same as Port Expander Library external dependencies.
Library Routines
SPI_Lcd_Config
Prototype |
void SPI_Lcd_Config(char DeviceAddress); |
---|---|
Description |
Initializes the Lcd module via SPI interface. |
Parameters |
|
Returns |
Nothing. |
Requires |
Global variables :
|
Example |
// Port Expander module connections sbit SPExpanderRST at LATF0_bit; sbit SPExpanderCS at LATF1_bit; sbit SPExpanderRST_Direction at TRISF0_bit; sbit SPExpanderCS_Direction at TRISF1_bit; // End Port Expander module connections // If Port Expander Library uses SPI1 module SPI1_Init(); // Initialize SPI module used with PortExpander SPI_Lcd_Config(0); // initialize Lcd over SPI interface |
Notes |
None. |
SPI_Lcd_Out
Prototype |
void SPI_Lcd_Out(char row, char column, char *text); |
---|---|
Description |
Prints text on the Lcd starting from specified position. Both string variables and literals can be passed as a text. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routine. |
Example |
// Write text "Hello!" on Lcd starting from row 1, column 3: SPI_Lcd_Out(1, 3, "Hello!"); |
Notes |
None. |
SPI_Lcd_Out_Cp
Prototype |
void SPI_Lcd_Out_CP(char *text); |
---|---|
Description |
Prints text on the Lcd at current cursor position. Both string variables and literals can be passed as a text. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routine. |
Example |
// Write text "Here!" at current cursor position: SPI_Lcd_Out_CP("Here!"); |
Notes |
None. |
SPI_Lcd_Chr
Prototype |
void SPI_Lcd_Chr(char Row, char Column, char Out_Char); |
---|---|
Description |
Prints character on Lcd at specified position. Both variables and literals can be passed as character. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routine. |
Example |
// Write character "i" at row 2, column 3: SPI_Lcd_Chr(2, 3, 'i'); |
Notes |
None. |
SPI_Lcd_Chr_Cp
Prototype |
void SPI_Lcd_Chr_CP(char Out_Char); |
---|---|
Description |
Prints character on Lcd at current cursor position. Both variables and literals can be passed as character. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routine. |
Example |
// Write character "e" at current cursor position: SPI_Lcd_Chr_Cp('e'); |
Notes |
None. |
SPI_Lcd_Cmd
Prototype |
void SPI_Lcd_Cmd(char out_char); |
---|---|
Description |
Sends command to Lcd. |
Parameters |
|
Returns |
Nothing. |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routine. |
Example |
// Clear Lcd display: SPI_Lcd_Cmd(_LCD_CLEAR); |
Notes |
Predefined constants can be passed to the routine, see Available SPI Lcd Commands. |
Available SPI Lcd Commands
SPI Lcd Command | Purpose |
---|---|
_LCD_FIRST_ROW | Move cursor to the 1st row |
_LCD_SECOND_ROW | Move cursor to the 2nd row |
_LCD_THIRD_ROW | Move cursor to the 3rd row |
_LCD_FOURTH_ROW | Move cursor to the 4th row |
_LCD_CLEAR | Clear display |
_LCD_RETURN_HOME | Return cursor to home position, returns a shifted display to its original position. Display data RAM is unaffected. |
_LCD_CURSOR_OFF | Turn off cursor |
_LCD_UNDERLINE_ON | Underline cursor on |
_LCD_BLINK_CURSOR_ON | Blink cursor on |
_LCD_MOVE_CURSOR_LEFT | Move cursor left without changing display data RAM |
_LCD_MOVE_CURSOR_RIGHT | Move cursor right without changing display data RAM |
_LCD_TURN_ON | Turn Lcd display on |
_LCD_TURN_OFF | Turn Lcd display off |
_LCD_SHIFT_LEFT | Shift display left without changing display data RAM |
_LCD_SHIFT_RIGHT | Shift display right without changing display data RAM |
Library Example
Default Pin Configuration
Use SPI_Lcd_Init
for default pin settings (see the first figure below).
char *text = "mikroElektronika";
// Port Expander module connections
sbit SPExpanderRST at LATD8_bit;
sbit SPExpanderCS at LATD9_bit;
sbit SPExpanderRST_Direction at TRISD8_bit;
sbit SPExpanderCS_Direction at TRISD9_bit;
// End Port Expander module connections
char i; // Loop variable
void Move_Delay() { // Function used for text moving
Delay_ms(500); // You can change the moving speed here
}
void main() {
CHECON = 0x32;
AD1PCFG = 0xFFFF; // Configure AN pins as digital
// If Port Expander Library uses SPI2 module
// Initialize SPI module used with PortExpander
SPI2_Init_Advanced(_SPI_MASTER,_SPI_8_BIT, 4, _SPI_SS_DISABLE,_SPI_DATA_SAMPLE_MIDDLE,_SPI_CLK_IDLE_LOW,_SPI_ACTIVE_2_IDLE);
SPI_Lcd_Config(0); // Initialize Lcd over SPI interface
SPI_Lcd_Cmd(_LCD_CLEAR); // Clear display
SPI_Lcd_Cmd(_LCD_CURSOR_OFF); // Turn cursor off
SPI_Lcd_Out(1,6, "mikroE"); // Print text to Lcd, 1st row, 6th column
SPI_Lcd_Chr_CP('!'); // Append '!'
SPI_Lcd_Out(2,1, text); // Print text to Lcd, 2nd row, 1st column
// SPI_Lcd_Out(3,1,"mikroE"); // For Lcd with more than two rows
// SPI_Lcd_Out(4,15,"mikroE"); // For Lcd with more than two rows
Delay_ms(2000);
// Moving text
for(i=0; i<4; i++) { // Move text to the right 4 times
SPI_Lcd_Cmd(_LCD_SHIFT_RIGHT);
Move_Delay();
}
while(1) { // Endless loop
for(i=0; i<8; i++) { // Move text to the left 7 times
SPI_Lcd_Cmd(_LCD_SHIFT_LEFT);
Move_Delay();
}
for(i=0; i<8; i++) { // Move text to the right 7 times
SPI_Lcd_Cmd(_LCD_SHIFT_RIGHT);
Move_Delay();
}
}
}
Lcd HW connection by default initialization (using SPI_Lcd_Init
)
What do you think about this topic ? Send us feedback!