SPI Lcd Library
The mikroC PRO for AVR provides a library for communication with Lcd (with HD44780 compliant controllers) in 4-bit mode via SPI interface.

- The library uses the SPI module for communication. The user must initialize the SPI module before using the SPI Lcd Library.
- This Library is designed to work with the mikroElektronika's Serial Lcd Adapter Board pinout. See schematic at the bottom of this page for details.
Library Dependency Tree
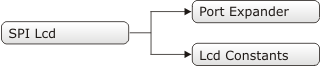
External dependencies of SPI Lcd Library
The implementation of SPI Lcd Library routines is based on Port Expander Library routines.
External dependencies are the same as Port Expander Library external dependencies.
Library Routines
SPI_Lcd_Config
Prototype |
void SPI_Lcd_Config(char DeviceAddress); |
---|---|
Returns |
Nothing. |
Description |
Initializes the Lcd module via SPI interface. Parameters :
|
Requires |
Global variables :
|
Example |
// Port Expander module connections sbit SPExpanderRST at PORTB0_bit; sbit SPExpanderCS at PORTB1_bit; sbit SPExpanderRST_Direction at DDB0_bit; sbit SPExpanderCS_Direction at DDB1_bit; // End Port Expander module connections void main() { // If Port Expander Library uses SPI1 module SPI1_Init(); // Initialize SPI module used with PortExpander SPI_Lcd_Config(0); // initialize Lcd over SPI interface |
SPI_Lcd_Out
Prototype |
void SPI_Lcd_Out(char row, char column, char *text); |
---|---|
Returns |
Nothing. |
Description |
Prints text on the Lcd starting from specified position. Both string variables and literals can be passed as a text. Parameters :
|
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routines. |
Example |
// Write text "Hello!" on Lcd starting from row 1, column 3: SPI_Lcd_Out(1, 3, "Hello!"); |
SPI_Lcd_Out_Cp
Prototype |
void SPI_Lcd_Out_CP(char *text); |
---|---|
Returns |
Nothing. |
Description |
Prints text on the Lcd at current cursor position. Both string variables and literals can be passed as a text. Parameters :
|
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routines. |
Example |
// Write text "Here!" at current cursor position: SPI_Lcd_Out_CP("Here!"); |
SPI_Lcd_Chr
Prototype |
void SPI_Lcd_Chr(char Row, char Column, char Out_Char); |
---|---|
Returns |
Nothing. |
Description |
Prints character on Lcd at specified position. Both variables and literals can be passed as character. Parameters :
|
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routines. |
Example |
// Write character "i" at row 2, column 3: SPI_Lcd_Chr(2, 3, 'i'); |
SPI_Lcd_Chr_Cp
Prototype |
void SPI_Lcd_Chr_CP(char Out_Char); |
---|---|
Returns |
Nothing. |
Description |
Prints character on Lcd at current cursor position. Both variables and literals can be passed as character. Parameters :
|
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routines. |
Example |
// Write character "e" at current cursor position: SPI_Lcd_Chr_Cp('e'); |
SPI_Lcd_Cmd
Prototype |
void SPI_Lcd_Cmd(char out_char); |
---|---|
Returns |
Nothing. |
Description |
Sends command to Lcd. Parameters :
![]() |
Requires |
Lcd needs to be initialized for SPI communication, see SPI_Lcd_Config routines. |
Example |
// Clear Lcd display: SPI_Lcd_Cmd(_LCD_CLEAR); |
Available SPI Lcd Commands
SPI Lcd Command | Purpose |
---|---|
_LCD_FIRST_ROW | Move cursor to the 1st row |
_LCD_SECOND_ROW | Move cursor to the 2nd row |
_LCD_THIRD_ROW | Move cursor to the 3rd row |
_LCD_FOURTH_ROW | Move cursor to the 4th row |
_LCD_CLEAR | Clear display |
_LCD_RETURN_HOME | Return cursor to home position, returns a shifted display to its original position. Display data RAM is unaffected. |
_LCD_CURSOR_OFF | Turn off cursor |
_LCD_UNDERLINE_ON | Underline cursor on |
_LCD_BLINK_CURSOR_ON | Blink cursor on |
_LCD_MOVE_CURSOR_LEFT | Move cursor left without changing display data RAM |
_LCD_MOVE_CURSOR_RIGHT | Move cursor right without changing display data RAM |
_LCD_TURN_ON | Turn Lcd display on |
_LCD_TURN_OFF | Turn Lcd display off |
_LCD_SHIFT_LEFT | Shift display left without changing display data RAM |
_LCD_SHIFT_RIGHT | Shift display right without changing display data RAM |
Library Example
This example demonstrates how to communicate Lcd via the SPI module, using serial to parallel convertor MCP23S17.
char *text = "mikroElektronika"; // Port Expander module connections sbit SPExpanderRST at PORTB0_bit; sbit SPExpanderCS at PORTB1_bit; sbit SPExpanderRST_Direction at DDB0_bit; sbit SPExpanderCS_Direction at DDB1_bit; // End Port Expander module connections void main() { // If Port Expander Library uses SPI1 module SPI1_Init(); // Initialize SPI module used with PortExpander // // If Port Expander Library uses SPI2 module // SPI2_Init(); // Initialize SPI module used with PortExpander SPI_Lcd_Config(0); // Initialize Lcd over SPI interface SPI_Lcd_Cmd(_LCD_CLEAR); // Clear display SPI_Lcd_Cmd(_LCD_CURSOR_OFF); // Turn cursor off SPI_Lcd_Out(1,6, "mikroE"); // Print text to Lcd, 1st row, 6th column SPI_Lcd_Chr_CP('!'); // Append '!' SPI_Lcd_Out(2,1, text); // Print text to Lcd, 2nd row, 1st column // SPI_Lcd_Out(3,1,"mikroE"); // For Lcd with more than two rows // SPI_Lcd_Out(4,15,"mikroE"); // For Lcd with more than two rows }
HW Connection
SPI Lcd HW connection
What do you think about this topic ? Send us feedback!